Spring @TestPropertySource example
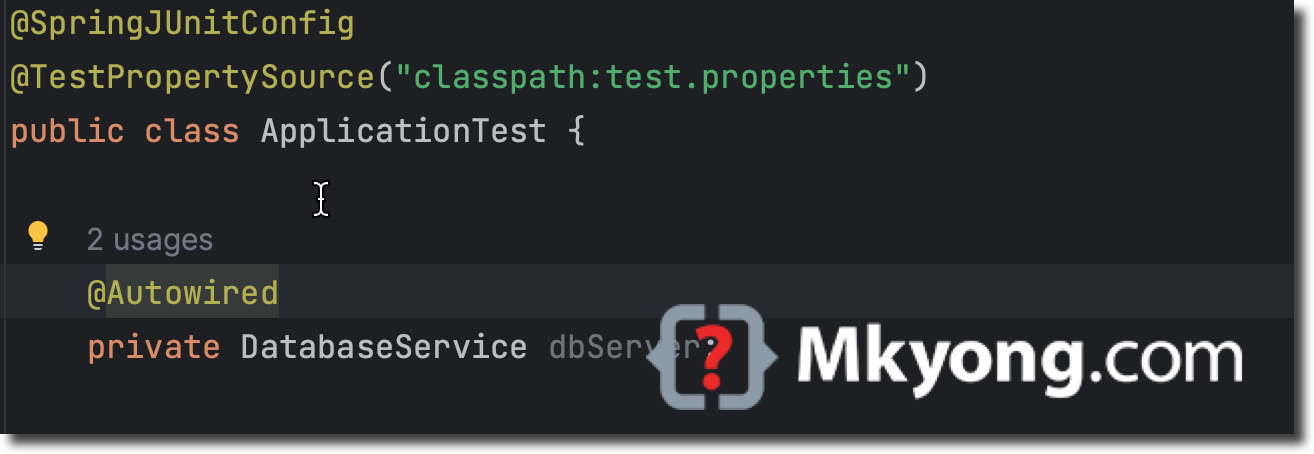
In Spring integration tests, we can use @TestPropertySource
to override the application properties which default loaded from application.properties
or application.yml
.
Table of contents
- 1. Service Class
- 2. Test with the @TestPropertySource
- 3. @TestPropertySource Inline Properties
- 4. Download Source Code
- 5. References
P.S Tested with Spring Boot 3.1.2
1. Service Class
1.1 We have a service class to get the values from the application.properties
.
# Database
db.name=mkyong
db.thread-pool=5
package com.mkyong.service;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
@Service
public class DatabaseService {
@Value("${db.name:hello}")
private String name;
@Value("${db.thread-pool:3}")
private Integer threadPool;
//getters, setters and etc.
}
1.2 Starts the Spring Boot application.
package com.mkyong;
import com.mkyong.service.DatabaseService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application implements CommandLineRunner {
@Autowired
private DatabaseService dbConfig;
@Override
public void run(String... args) throws Exception {
System.out.println(dbConfig);
}
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Output
DatabaseService{name='mkyong', threadPool=5}
2. Test with the @TestPropertySource
The @SpringBootTest
loads the property values from the following sequence:
- Properties from
@TestPropertySource
- The
application.properties
orapplication.yml
from thesrc/test/resources
directory. - The
application.properties
orapplication.yml
from thesrc/main/resources
directory.
Review the project structure.
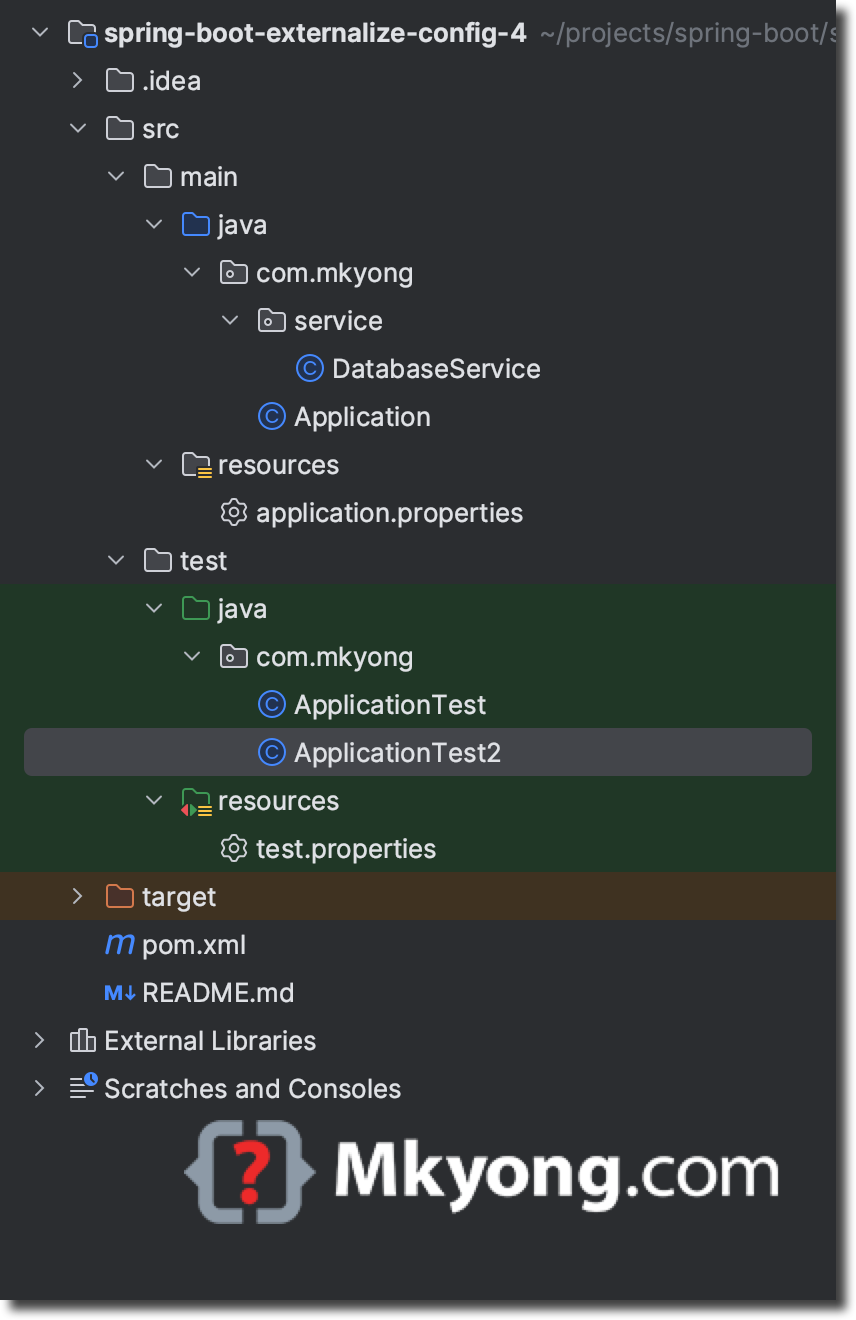
Spring loads the property values from the src/main/resources/application.properties
in this case.
# Database
db.name=mkyong
db.thread-pool=5
We create a new src/test/resources/test.properties
with new thread pool values for the tests.
db.thread-pool=10
And we use @TestPropertySource
to load the test.properties
file and override the thread pool values from the main application’s property value.
package com.mkyong;
import com.mkyong.service.DatabaseService;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.TestPropertySource;
import static org.junit.jupiter.api.Assertions.assertEquals;
@SpringBootTest
@TestPropertySource("classpath:test.properties")
public class ApplicationTest2 {
@Autowired
private DatabaseService dbServer;
// This value from the application.properties.
@Test
public void testDatabaseName() {
assertEquals("mkyong", dbServer.getName());
}
// We expect 10 from the test.properties; the original application.properties is 5.
@Test
public void testDatabaseThreadPool() {
assertEquals(10, dbServer.getThreadPool());
}
}
3. @TestPropertySource Inline Properties
The @TestPropertySource
supports inline properties directly.
// inline properties
@TestPropertySource(properties = {"db.thread-pool=10"})
We can also defined multiple inline properties directly,
//multiple inline properties
@TestPropertySource(properties = {
"db.thread-pool=10",
"db.name=mkyong"
})
4. Download Source Code
$ git clone https://github.com/mkyong/spring-boot.git
$ cd spring-boot-externalize-config-4
$ mvn test
$ mvn spring-boot:run
application.yml is not supported