Spring Boot Hello World Example
In this article, we will show you a simple Spring Boot web application, publish an endpoint /
, access it, and the endpoint /
will return a String hello world
as a result.
Technologies used:
- Spring Boot 3.1.2
- Java 17
- Maven 3.8.6
Table of contents:
- 1. Project Directory
- 2. Spring Boot Maven Dependencies
- 3. Project Dependencies in Tree Structure
- 4. Spring Boot Web Application
- 5. Spring Boot Entry Point
- 6. Add Unit Test
- 7. Demo
- 8. Create an executable JAR
- 9. Download Source Code
- 10. References
Note
Spring Boot 3.x requires Java 17.
1. Project Directory
A standard Maven folder Structure.
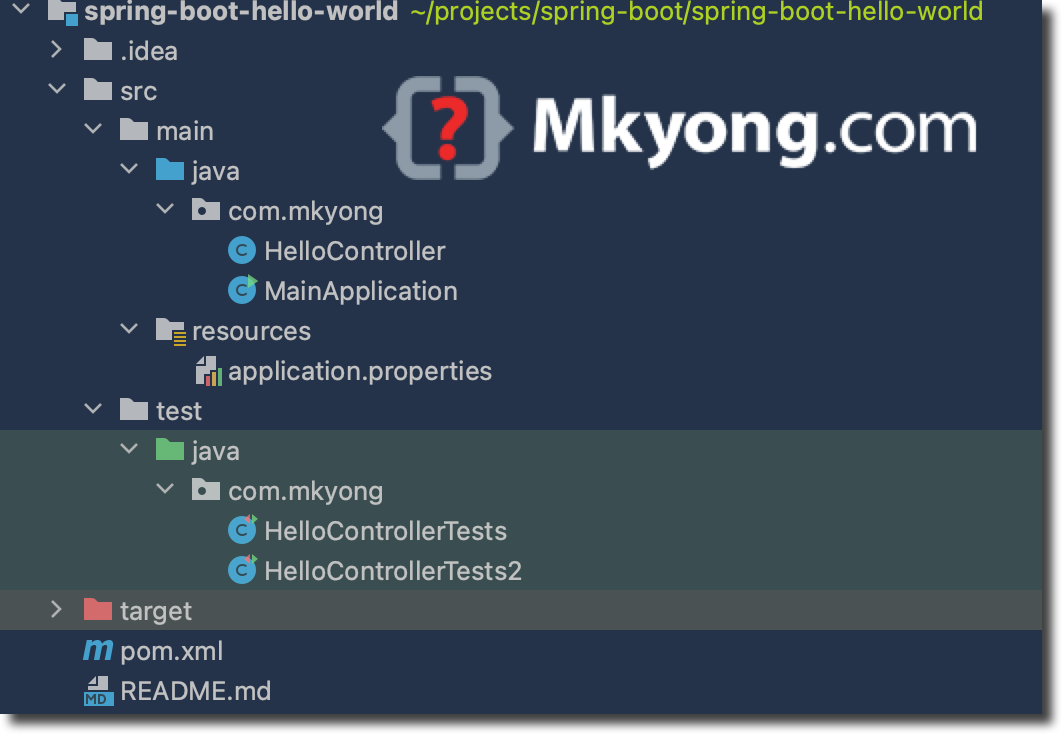
2. Spring Boot Maven Dependencies
The spring-boot-starter-parent
provide a base version for the Spring Boot applications. To develop web-related services, put spring-boot-starter-web
.
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.1.2</version>
<relativePath/> <!-- lookup parent from repository, not local -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Below is the complete pom.xml
.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<artifactId>spring-boot-hello-world</artifactId>
<packaging>jar</packaging>
<name>Spring Boot Hello World Example</name>
<version>1.0</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.1.2</version>
<relativePath/> <!-- lookup parent from repository, not local -->
</parent>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.11.0</version>
<configuration>
<source>${java.version}</source>
<target>${java.version}</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
3. Project Dependencies in Tree Structure
For Spring Boot 3, the spring-boot-starter-web
bring in the following main dependencies:
- Spring boot configuration stuff.
- Spring web mvc components.
- Embedded Tomcat 10.1.11.
- Slf4j logback for logging.
- Snakeyaml for external YAML properties.
- Jackson 2.x for JSON binding.
In Maven, we can use mvn dependency:tree
to review the project dependencies in a tree structure.
$ mvn dependency:tree
[INFO] org.springframework.boot:spring-boot-hello-world:jar:1.0
[INFO] +- org.springframework.boot:spring-boot-starter-web:jar:3.1.2:compile
[INFO] | +- org.springframework.boot:spring-boot-starter:jar:3.1.2:compile
[INFO] | | +- org.springframework.boot:spring-boot:jar:3.1.2:compile
[INFO] | | +- org.springframework.boot:spring-boot-autoconfigure:jar:3.1.2:compile
[INFO] | | +- org.springframework.boot:spring-boot-starter-logging:jar:3.1.2:compile
[INFO] | | | +- ch.qos.logback:logback-classic:jar:1.4.8:compile
[INFO] | | | | \- ch.qos.logback:logback-core:jar:1.4.8:compile
[INFO] | | | +- org.apache.logging.log4j:log4j-to-slf4j:jar:2.20.0:compile
[INFO] | | | | \- org.apache.logging.log4j:log4j-api:jar:2.20.0:compile
[INFO] | | | \- org.slf4j:jul-to-slf4j:jar:2.0.7:compile
[INFO] | | +- jakarta.annotation:jakarta.annotation-api:jar:2.1.1:compile
[INFO] | | \- org.yaml:snakeyaml:jar:1.33:compile
[INFO] | +- org.springframework.boot:spring-boot-starter-json:jar:3.1.2:compile
[INFO] | | +- com.fasterxml.jackson.core:jackson-databind:jar:2.15.2:compile
[INFO] | | | +- com.fasterxml.jackson.core:jackson-annotations:jar:2.15.2:compile
[INFO] | | | \- com.fasterxml.jackson.core:jackson-core:jar:2.15.2:compile
[INFO] | | +- com.fasterxml.jackson.datatype:jackson-datatype-jdk8:jar:2.15.2:compile
[INFO] | | +- com.fasterxml.jackson.datatype:jackson-datatype-jsr310:jar:2.15.2:compile
[INFO] | | \- com.fasterxml.jackson.module:jackson-module-parameter-names:jar:2.15.2:compile
[INFO] | +- org.springframework.boot:spring-boot-starter-tomcat:jar:3.1.2:compile
[INFO] | | +- org.apache.tomcat.embed:tomcat-embed-core:jar:10.1.11:compile
[INFO] | | +- org.apache.tomcat.embed:tomcat-embed-el:jar:10.1.11:compile
[INFO] | | \- org.apache.tomcat.embed:tomcat-embed-websocket:jar:10.1.11:compile
[INFO] | +- org.springframework:spring-web:jar:6.0.11:compile
[INFO] | | +- org.springframework:spring-beans:jar:6.0.11:compile
[INFO] | | \- io.micrometer:micrometer-observation:jar:1.11.2:compile
[INFO] | | \- io.micrometer:micrometer-commons:jar:1.11.2:compile
[INFO] | \- org.springframework:spring-webmvc:jar:6.0.11:compile
[INFO] | +- org.springframework:spring-aop:jar:6.0.11:compile
[INFO] | +- org.springframework:spring-context:jar:6.0.11:compile
[INFO] | \- org.springframework:spring-expression:jar:6.0.11:compile
[INFO] \- org.springframework.boot:spring-boot-starter-test:jar:3.1.2:test
[INFO] +- org.springframework.boot:spring-boot-test:jar:3.1.2:test
[INFO] +- org.springframework.boot:spring-boot-test-autoconfigure:jar:3.1.2:test
[INFO] +- com.jayway.jsonpath:json-path:jar:2.8.0:test
[INFO] | \- org.slf4j:slf4j-api:jar:2.0.7:compile
[INFO] +- jakarta.xml.bind:jakarta.xml.bind-api:jar:4.0.0:test
[INFO] | \- jakarta.activation:jakarta.activation-api:jar:2.1.2:test
[INFO] +- net.minidev:json-smart:jar:2.4.11:test
[INFO] | \- net.minidev:accessors-smart:jar:2.4.11:test
[INFO] | \- org.ow2.asm:asm:jar:9.3:test
[INFO] +- org.assertj:assertj-core:jar:3.24.2:test
[INFO] | \- net.bytebuddy:byte-buddy:jar:1.14.5:test
[INFO] +- org.hamcrest:hamcrest:jar:2.2:test
[INFO] +- org.junit.jupiter:junit-jupiter:jar:5.9.3:test
[INFO] | +- org.junit.jupiter:junit-jupiter-api:jar:5.9.3:test
[INFO] | | +- org.opentest4j:opentest4j:jar:1.2.0:test
[INFO] | | +- org.junit.platform:junit-platform-commons:jar:1.9.3:test
[INFO] | | \- org.apiguardian:apiguardian-api:jar:1.1.2:test
[INFO] | +- org.junit.jupiter:junit-jupiter-params:jar:5.9.3:test
[INFO] | \- org.junit.jupiter:junit-jupiter-engine:jar:5.9.3:test
[INFO] | \- org.junit.platform:junit-platform-engine:jar:1.9.3:test
[INFO] +- org.mockito:mockito-core:jar:5.3.1:test
[INFO] | +- net.bytebuddy:byte-buddy-agent:jar:1.14.5:test
[INFO] | \- org.objenesis:objenesis:jar:3.3:test
[INFO] +- org.mockito:mockito-junit-jupiter:jar:5.3.1:test
[INFO] +- org.skyscreamer:jsonassert:jar:1.5.1:test
[INFO] | \- com.vaadin.external.google:android-json:jar:0.0.20131108.vaadin1:test
[INFO] +- org.springframework:spring-core:jar:6.0.11:compile
[INFO] | \- org.springframework:spring-jcl:jar:6.0.11:compile
[INFO] +- org.springframework:spring-test:jar:6.0.11:test
[INFO] \- org.xmlunit:xmlunit-core:jar:2.9.1:test
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 5.728 s
[INFO] Finished at: 2023-08-15T14:57:04+08:00
[INFO] ------------------------------------------------------------------------
4. Spring Boot Web Application
The @RestController
annotation is the controller to handle incoming HTTP web requests, equivalent to @Controller
and @ResponseBody
. The @RequestMapping("/")
annotation is for routing; it mapped the HTTP web request with a /
path to the hello()
method.
package com.mkyong;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
// @Controller
// @ResponseBody
@RestController
public class HelloController {
@RequestMapping("/")
String hello() {
return "Hello World, Spring Boot!";
}
}
5. Spring Boot Entry Point
Create a @SpringBootApplication
annotated class, which is the main entry point for the Spring boot application. Run this class, and it will start the entire Spring Boot web application.
package com.mkyong;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MainApplication {
public static void main(String[] args) {
SpringApplication.run(MainApplication.class, args);
}
}
6. Add Unit Test
Below are two examples of writing unit tests for the spring boot web applications.
6.1 MockMvc
We can use MockMvc
to perform the test on the path /
. The MockMvc
mocks all MVC components; it’s fast because there is no need to start an entire HTTP server.
package com.mkyong;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.MediaType;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import static org.hamcrest.Matchers.equalTo;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.content;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@SpringBootTest
@AutoConfigureMockMvc
public class HelloControllerTests {
@Autowired
private MockMvc mvc;
@Test
public void welcome_ok() throws Exception {
mvc.perform(MockMvcRequestBuilders.get("/").accept(MediaType.APPLICATION_JSON))
.andExpect(status().isOk())
.andExpect(content().string(equalTo("Hello World, Spring Boot!")));
}
}
6.2 TestRestTemplate
Alternatively, we can use TestRestTemplate
to perform the same test. It requires a running server, usually with a random port, and making actual HTTP requests to the running server.
package com.mkyong;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.web.client.TestRestTemplate;
import org.springframework.http.ResponseEntity;
import static org.assertj.core.api.AssertionsForClassTypes.assertThat;
//@SpringBootTest(classes = MainApplication.class, webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class HelloControllerTests2 {
@Autowired
private TestRestTemplate template;
@Test
public void hello_ok() throws Exception {
ResponseEntity<String> response = template.getForEntity("/", String.class);
assertThat(response.getBody()).isEqualTo("Hello World, Spring Boot!");
}
}
7. Demo
Navigate to the project folder and type mvn spring-boot:run
to run the spring boot application.
$ mvn spring-boot:run
By default, Spring Boot starts a self-embedded Tomcat at port 8080 and deploys the spring boot web application.
$ mvn spring-boot:run
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v3.1.2)
com.mkyong.MainApplication : Starting MainApplication using Java 17 with PID 53029 (...)
com.mkyong.MainApplication : No active profile set, falling back to 1 default profile: "default"
o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
o.apache.catalina.core.StandardService : Starting service [Tomcat]
o.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/10.1.11]
o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 487 ms
o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
com.mkyong.MainApplication : Started MainApplication in 0.923 seconds (process running for 1.105)
P.S We can press ctrl + c
to exit the Spring Boot application.
We can use curl
to send an HTTP request to the /
endpoint.
curl localhost:8080
Hello World, Spring Boot!
Or you can open a browser and access the http://localhost:8080/
; the browser will return a plain text Hello World, Spring Boot!
.
8. Create an executable JAR
8.1 In pom.xml
, the packaging
is jar
; it tells Maven to package the project as a jar
file.
<artifactId>spring-boot-hello-world</artifactId>
<packaging>jar</packaging>
<name>Spring Boot Hello World Example</name>
<version>1.0</version>
And the spring-boot-maven-plugin allows Maven easier to package the Spring Boot project as an executable jar file.
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
8.2 A standard mvn package
will compile and pack everything into an executable JAR file.
$ cd [project-path]
$ mvn clean package
$ java -jar target/spring-boot-hello-world-1.0.jar
9. Download Source Code
$ git clone https://github.com/mkyong/spring-boot.git
$ cd spring-boot-hello-world
$ mvn spring-boot:run
Wonderful sir for starters.
I am looking for springBoot with microservice sample and simple example to start up
good content
Thank You