How to access values from application.properties in Spring Boot
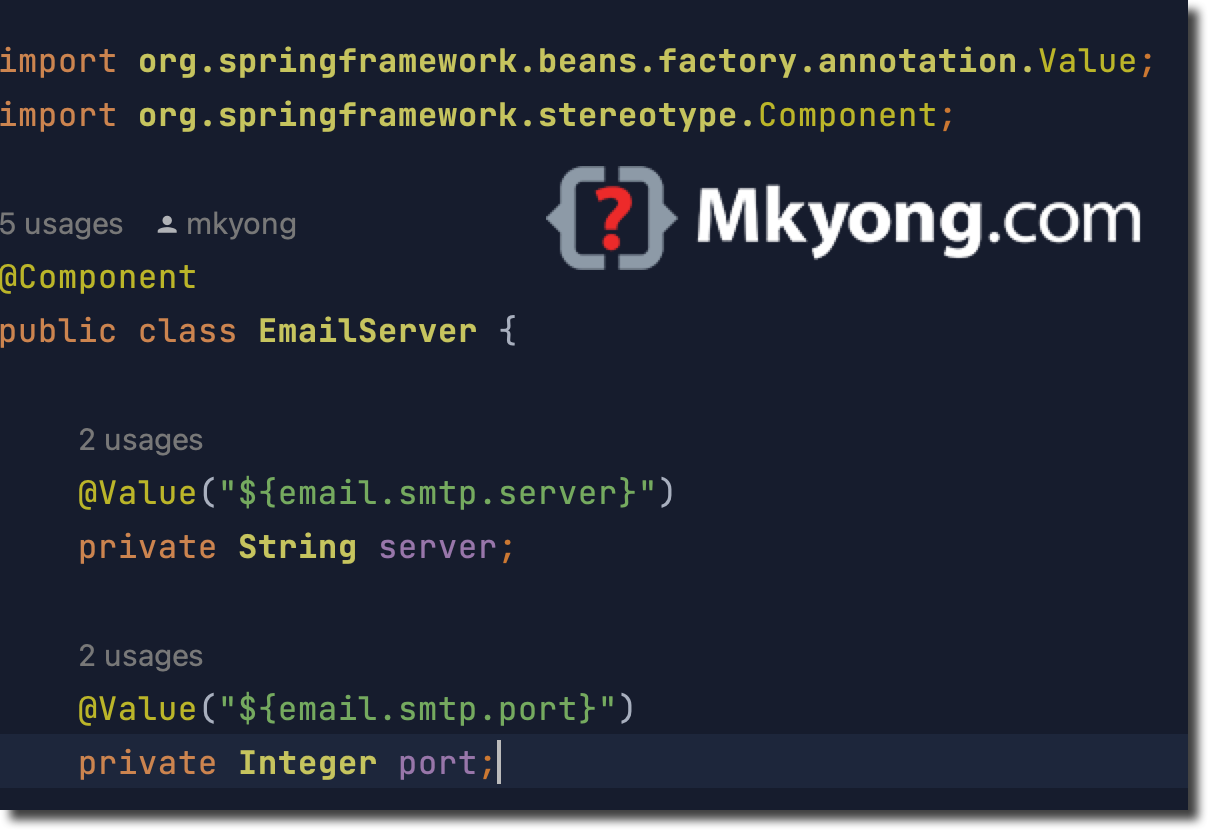
In Spring Boot, we can use @Value
annotation to access the values defined in the application.properties
from Spring-managed beans.
#Logging
logging.level.org.springframework.web=ERROR
logging.level.com.mkyong=DEBUG
email.smtp.server=smtp.gmail.com
import org.springframework.beans.factory.annotation.Value;
@Value("${email.smtp.server}")
private String server;
Table of contents:
- 1. application.properties
- 1. @Value
- 2. Environment
- 3. @ConfigurationProperties
- 4. Download Source Code
- 5. References
P.S. Tested with Spring Boot 3.1.2
1. application.properties
Spring default loads the application.properties
file from the root classpath. Later, we will show a few ways to access the values defined in the below application.properties
.
#Logging
logging.level.org.springframework.web=ERROR
logging.level.com.mkyong=DEBUG
#email server
email.smtp.server=smtp.gmail.com
email.smtp.port=467
[email protected]
Here’s the project structure for reference.
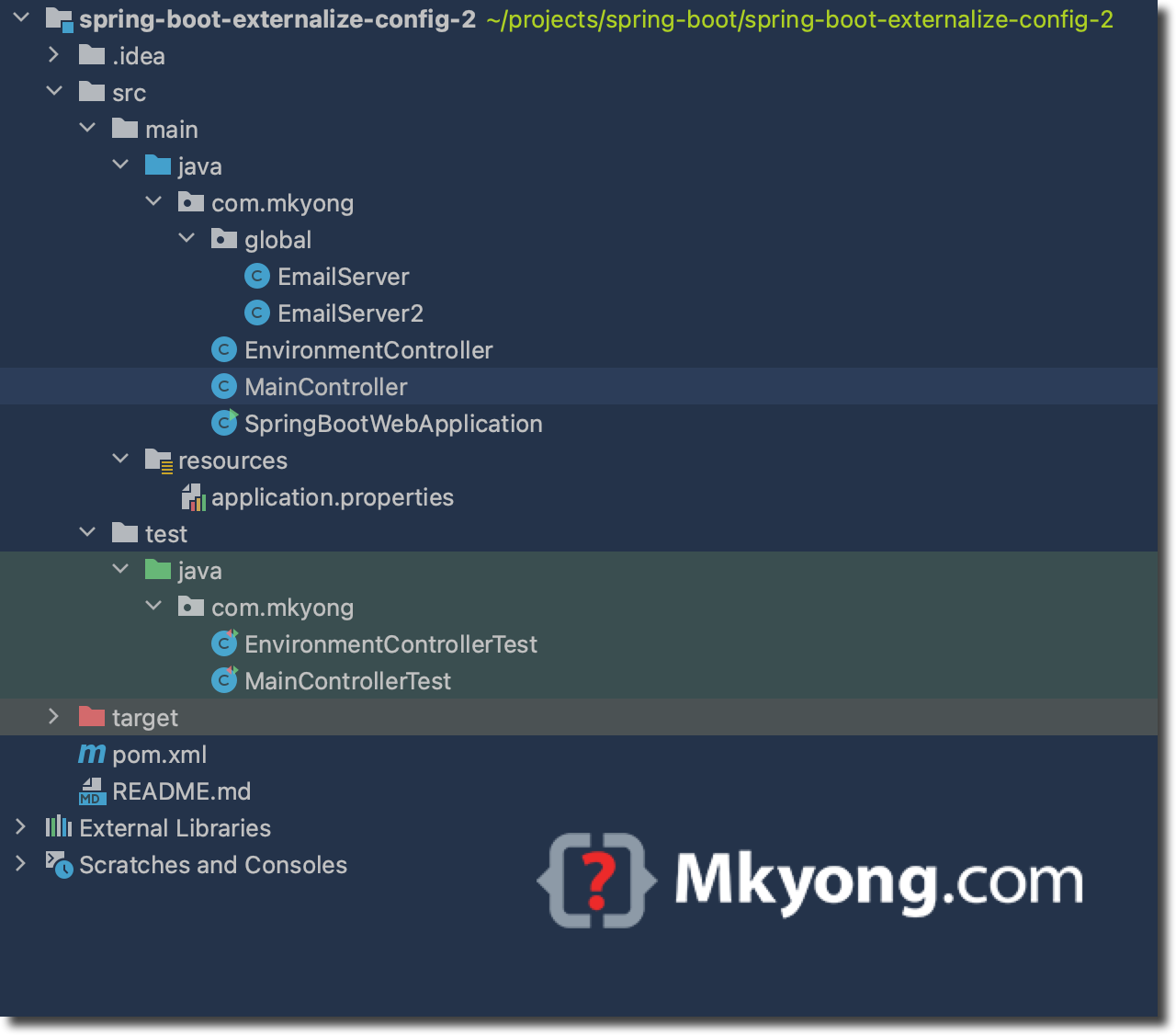
1. @Value
In Spring managed beans, we can use the @Value
annotation to access the values defined in the application.properties
.
package com.mkyong.global;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class EmailServer {
// injects smtp.gmail.com
@Value("${email.smtp.server}")
private String server;
// injects 467
@Value("${email.smtp.port}")
private Integer port;
// injects [email protected]
@Value("${email.smtp.username}")
private String username;
//setters, getters
}
Below is a REST controller to return the above EmailServer
object in JSON format.
package com.mkyong;
import com.mkyong.global.EmailServer;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MainController {
@Autowired
private EmailServer email;
@GetMapping("/email")
public EmailServer getEmail() {
return email;
}
}
2. Environment
Alternatively, we can also auto-wire the org.springframework.core.env.Environment
bean and access the values defined in the application.properties
.
package com.mkyong;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.HashMap;
import java.util.Map;
@RestController
public class EnvironmentController {
@Autowired
private Environment env;
@GetMapping("/env")
public Map<String, String> getEmailFromEnvironment() {
String server = env.getProperty("email.smtp.server");
String username = env.getProperty("email.smtp.username");
String port = env.getProperty("email.smtp.port");
Map<String, String> map = new HashMap<>();
map.put("server", server);
map.put("username", username);
map.put("port", port);
return map;
}
}
3. @ConfigurationProperties
The @ConfigurationProperties
bind values from application.properties
to a Java object.
package com.mkyong.global;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties("email.smtp") // prefix
public class EmailServer2 {
private String server; // injects smtp.gmail.com
private Integer port; // injects 467
private String username; // injects [email protected]
//getters, setters
}
4. Download Source Code
$ git clone https://github.com/mkyong/spring-boot.git
$ cd spring-boot-externalize-config-2
$ mvn test
$ mvn spring-boot:run
$ curl localhost:8080/email
$ curl localhost:8080/email2
$ curl localhost:8080/env
Can you please share if there is a way to iterate through all properties defined in application.properties, thank you