Maven + Quarkus Hello World example
This article shows how to use the quarkus-maven-plugin to create or scaffold a Maven + Quarkus JAX-RS hello world project.
Technologies used:
- Quarkus 1.11.0
- Maven 3.6.3
- Java 11
Topics
- Creating a new Maven + Quartus project
- Maven + Quarkus Project Structure
- Quarkus and pom.xml
- Quarkus Dependencies
- Quarkus JAX-RX endpoint
- Quarkus and Dockerfile
- Run Quarkus in Dev Mode
- Download Source Code
- References
1. Creating a new Maven + Quartus project
We can use the quarkus-maven-plugin to create a Quarkus JAX-RS project.
1.1 For Linux and macOS system.
mvn io.quarkus:quarkus-maven-plugin:1.11.0.Final:create \
-DprojectGroupId=com.mkyong \
-DprojectArtifactId=quarkus-hello-world-maven \
-DclassName="com.mkyong.quarkus.HelloResource" \
-Dpath="/hello"
1.2 For Windows, cmd
or command prompt, replace the new line \
with a ^
.
mvn io.quarkus:quarkus-maven-plugin:1.11.0.Final:create ^
-DprojectGroupId=com.mkyong ^
-DprojectArtifactId=quarkus-hello-world-maven ^
-DclassName="com.mkyong.quarkus.HelloResource" ^
-Dpath="/hello"
Output
-----------
[INFO]
[INFO] ========================================================================================
[INFO] Your new application has been created in C:\Users\mkyong\projects\quarkus\quarkus-hello-world-maven
[INFO] Navigate into this directory and launch your application with mvn quarkus:dev
[INFO] Your application will be accessible on http://localhost:8080
[INFO] ========================================================================================
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 2.774 s
[INFO] Finished at: 2021-01-26T13:25:14+08:00
[INFO] ------------------------------------------------------------------------
2. Maven + Quarkus Project Structure
The above command created a new project named quarkus-hello-world-maven
, and generates the following files and directories:
- Standard Maven directory structure.
- Maven
pom.xml
and related wrapper. HelloResource.java
for/hello
endpoint.- Unit Tests.
- A landing page
resources\META-INF\resources\index.html
forhttp://localhost:8080
Dockerfile
files, forjvm
ornative
mode.- The application configuration file,
application.properties
.
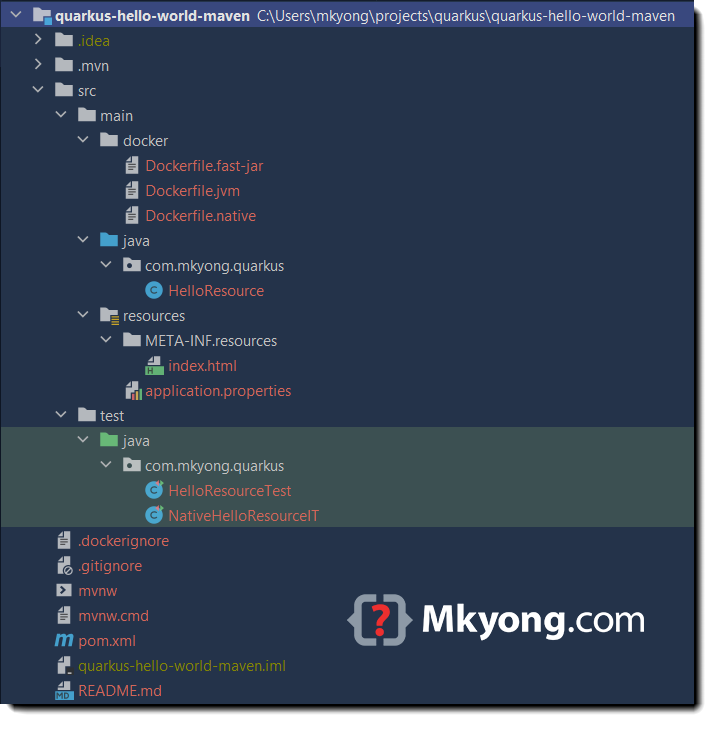
> cd quarkus-hello-world-maven
C:\Users\mkyong\projects\quarkus\quarkus-hello-world-maven> tree /F
Folder PATH listing
Volume serial number is A446-18E6
C:.
│ .dockerignore
│ .gitignore
│ mvnw
│ mvnw.cmd
│ pom.xml
│ README.md
│
├───.idea
│ misc.xml
│ vcs.xml
│ workspace.xml
│
├───.mvn
│ └───wrapper
│ maven-wrapper.properties
│ MavenWrapperDownloader.java
│
└───src
├───main
│ ├───docker
│ │ Dockerfile.fast-jar
│ │ Dockerfile.jvm
│ │ Dockerfile.native
│ │
│ ├───java
│ │ └───com
│ │ └───mkyong
│ │ └───quarkus
│ │ HelloResource.java
│ │
│ └───resources
│ │ application.properties
│ │
│ └───META-INF
│ └───resources
│ index.html
│
└───test
└───java
└───com
└───mkyong
└───quarkus
HelloResourceTest.java
NativeHelloResourceIT.java
3. Quarkus and pom.xml
Review the generated pom.xml
file.
3.1 The Quarkus JAX-RS project required only two dependencies: quarkus-arc
and quarkus-resteasy
. (The transitive dependencies are huge, read below for full Quarkus dependencies).
<dependencies>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-arc</artifactId>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-resteasy</artifactId>
</dependency>
</dependencies>
The Quarkus uses JUnit 5 and REST-assured for unit tests.
<dependencies>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-junit5</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
A complete pom.xml
.
<?xml version="1.0"?>
<project xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
https://maven.apache.org/xsd/maven-4.0.0.xsd"
xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mkyong</groupId>
<artifactId>quarkus-hello-world-maven</artifactId>
<version>1.0.0-SNAPSHOT</version>
<properties>
<surefire-plugin.version>2.22.1</surefire-plugin.version>
<maven.compiler.target>11</maven.compiler.target>
<quarkus.platform.version>1.11.0.Final</quarkus.platform.version>
<maven.compiler.source>11</maven.compiler.source>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<quarkus.platform.artifact-id>quarkus-universe-bom</quarkus.platform.artifact-id>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<maven.compiler.parameters>true</maven.compiler.parameters>
<quarkus-plugin.version>1.11.0.Final</quarkus-plugin.version>
<compiler-plugin.version>3.8.1</compiler-plugin.version>
<quarkus.platform.group-id>io.quarkus</quarkus.platform.group-id>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>${quarkus.platform.group-id}</groupId>
<artifactId>${quarkus.platform.artifact-id}</artifactId>
<version>${quarkus.platform.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-arc</artifactId>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-resteasy</artifactId>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-junit5</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-maven-plugin</artifactId>
<version>${quarkus-plugin.version}</version>
<extensions>true</extensions>
<executions>
<execution>
<goals>
<goal>build</goal>
<goal>generate-code</goal>
<goal>generate-code-tests</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>${compiler-plugin.version}</version>
</plugin>
<plugin>
<artifactId>maven-surefire-plugin</artifactId>
<version>${surefire-plugin.version}</version>
<configuration>
<systemPropertyVariables>
<java.util.logging.manager>org.jboss.logmanager.LogManager</java.util.logging.manager>
<maven.home>${maven.home}</maven.home>
</systemPropertyVariables>
</configuration>
</plugin>
</plugins>
</build>
<profiles>
<profile>
<id>native</id>
<activation>
<property>
<name>native</name>
</property>
</activation>
<build>
<plugins>
<plugin>
<artifactId>maven-failsafe-plugin</artifactId>
<version>${surefire-plugin.version}</version>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
<configuration>
<systemPropertyVariables>
<native.image.path>${project.build.directory}/${project.build.finalName}-runner</native.image.path>
<java.util.logging.manager>org.jboss.logmanager.LogManager</java.util.logging.manager>
<maven.home>${maven.home}</maven.home>
</systemPropertyVariables>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
<properties>
<quarkus.package.type>native</quarkus.package.type>
</properties>
</profile>
</profiles>
</project>
4. Quarkus Dependencies
4.1 Review the full project dependencies for a default Quarkus JAX-RS project.
The Quarkus core Technologies:
- Quarkus uses Vert.x and Netty at its core, providing reactive and non-blocking HTTP layer.
- Quarkus uses RESTEasy for the JAX-RS endpoint.
- Quarkus uses ArC as the dependency injection solution, which is based on the Contexts and Dependency Injection for Java 2.0 specification. The ArC is a subset of the CDI tailored for Quarkus’ architecture.
- Quarkus uses JBoss Log Manager as the default logging framework.
- Quartus uses a modified version of Undertow (Servlet/Websocket engine) that runs on top of Vert.x
- Quarkus uses SmallRye Config, implementation of Eclipse MicroProfile Config, for configuration data.
For testing:
- Quarkus uses JUnit 5 for testing.
- Quarkus uses rest-assured to test HTTP endpoints.
4.2 Project dependencies view on IntelliJ IDEA.
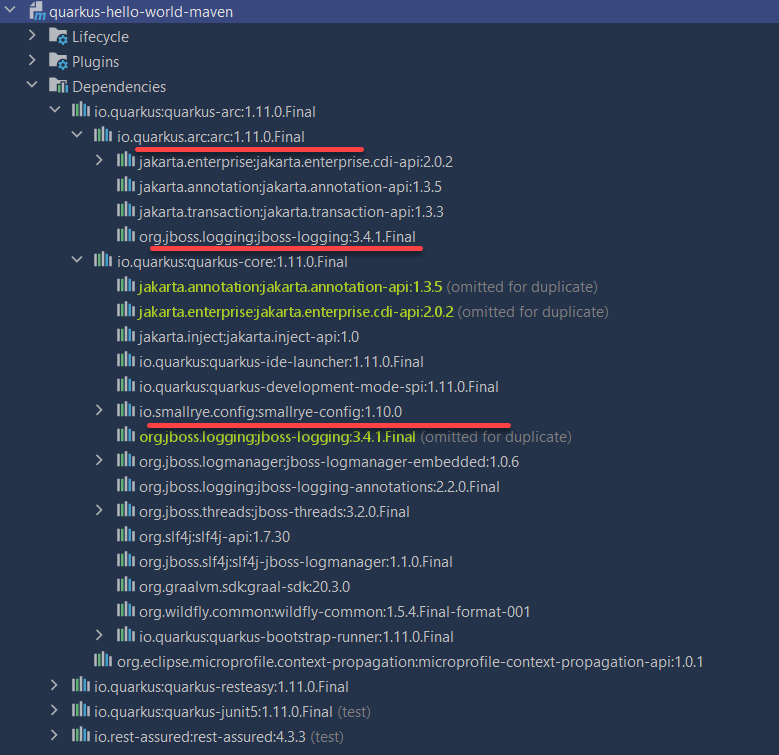
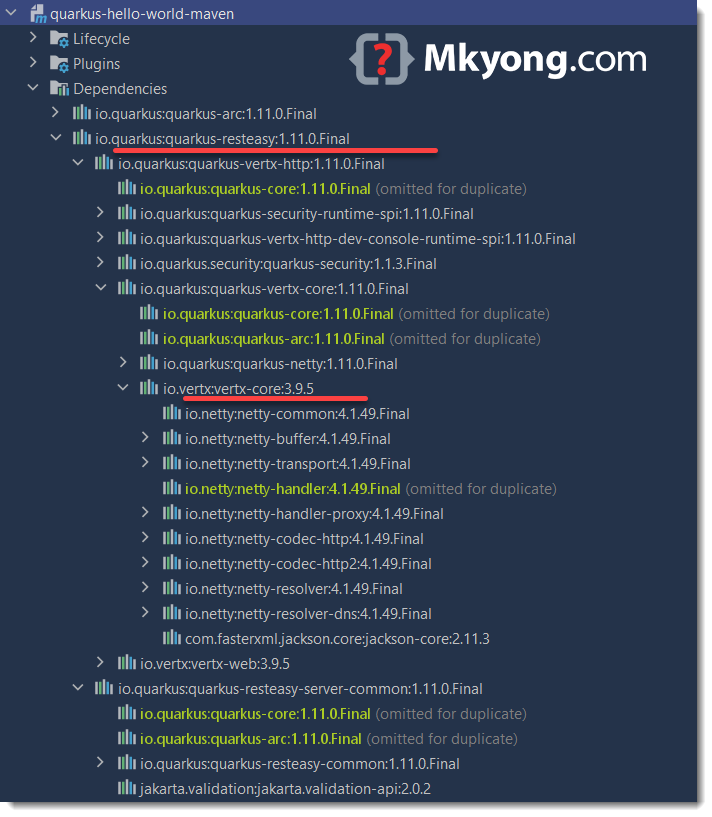
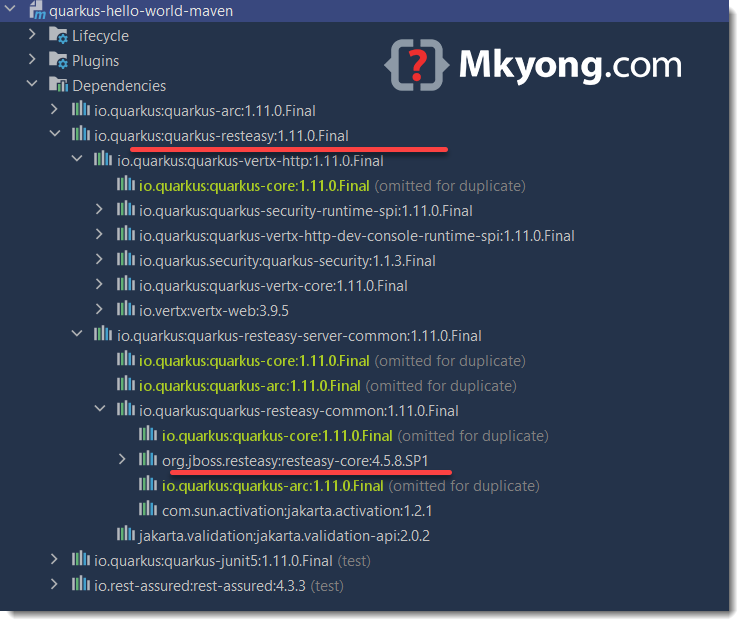
4.3 Maven command dependency:tree
to review the full project dependencies (a long list) in text format.
C:\Users\mkyong\projects\quarkus\quarkus-hello-world-maven>mvn dependency:tree
[INFO] Scanning for projects...
[INFO]
[INFO] ----------------< com.mkyong:quarkus-hello-world-maven >----------------
[INFO] Building quarkus-hello-world-maven 1.0.0-SNAPSHOT
[INFO] --------------------------------[ jar ]---------------------------------
[INFO]
[INFO] --- maven-dependency-plugin:2.8:tree (default-cli) @ quarkus-hello-world-maven ---
[INFO] com.mkyong:quarkus-hello-world-maven:jar:1.0.0-SNAPSHOT
[INFO] +- io.quarkus:quarkus-arc:jar:1.11.0.Final:compile
[INFO] | +- io.quarkus.arc:arc:jar:1.11.0.Final:compile
[INFO] | | +- jakarta.enterprise:jakarta.enterprise.cdi-api:jar:2.0.2:compile
[INFO] | | | +- jakarta.el:jakarta.el-api:jar:3.0.3:compile
[INFO] | | | \- jakarta.interceptor:jakarta.interceptor-api:jar:1.2.5:compile
[INFO] | | +- jakarta.annotation:jakarta.annotation-api:jar:1.3.5:compile
[INFO] | | +- jakarta.transaction:jakarta.transaction-api:jar:1.3.3:compile
[INFO] | | \- org.jboss.logging:jboss-logging:jar:3.4.1.Final:compile
[INFO] | +- io.quarkus:quarkus-core:jar:1.11.0.Final:compile
[INFO] | | +- jakarta.inject:jakarta.inject-api:jar:1.0:compile
[INFO] | | +- io.quarkus:quarkus-ide-launcher:jar:1.11.0.Final:compile
[INFO] | | +- io.quarkus:quarkus-development-mode-spi:jar:1.11.0.Final:compile
[INFO] | | +- io.smallrye.config:smallrye-config:jar:1.10.0:compile
[INFO] | | | +- io.smallrye.common:smallrye-common-annotation:jar:1.5.0:compile
[INFO] | | | +- io.smallrye.config:smallrye-config-common:jar:1.10.0:compile
[INFO] | | | | \- org.eclipse.microprofile.config:microprofile-config-api:jar:1.4:compile
[INFO] | | | +- io.smallrye.common:smallrye-common-expression:jar:1.5.0:compile
[INFO] | | | | \- io.smallrye.common:smallrye-common-function:jar:1.5.0:compile
[INFO] | | | +- io.smallrye.common:smallrye-common-constraint:jar:1.5.0:compile
[INFO] | | | \- io.smallrye.common:smallrye-common-classloader:jar:1.5.0:compile
[INFO] | | +- org.jboss.logmanager:jboss-logmanager-embedded:jar:1.0.6:compile
[INFO] | | +- org.jboss.logging:jboss-logging-annotations:jar:2.2.0.Final:compile
[INFO] | | +- org.jboss.threads:jboss-threads:jar:3.2.0.Final:compile
[INFO] | | +- org.slf4j:slf4j-api:jar:1.7.30:compile
[INFO] | | +- org.jboss.slf4j:slf4j-jboss-logmanager:jar:1.1.0.Final:compile
[INFO] | | +- org.graalvm.sdk:graal-sdk:jar:20.3.0:compile
[INFO] | | +- org.wildfly.common:wildfly-common:jar:1.5.4.Final-format-001:compile
[INFO] | | \- io.quarkus:quarkus-bootstrap-runner:jar:1.11.0.Final:compile
[INFO] | \- org.eclipse.microprofile.context-propagation:microprofile-context-propagation-api:jar:1.0.1:compile
[INFO] +- io.quarkus:quarkus-resteasy:jar:1.11.0.Final:compile
[INFO] | +- io.quarkus:quarkus-vertx-http:jar:1.11.0.Final:compile
[INFO] | | +- io.quarkus:quarkus-security-runtime-spi:jar:1.11.0.Final:compile
[INFO] | | +- io.quarkus:quarkus-vertx-http-dev-console-runtime-spi:jar:1.11.0.Final:compile
[INFO] | | +- io.quarkus.security:quarkus-security:jar:1.1.3.Final:compile
[INFO] | | | \- io.smallrye.reactive:mutiny:jar:0.12.5:compile
[INFO] | | | \- org.reactivestreams:reactive-streams:jar:1.0.3:compile
[INFO] | | +- io.quarkus:quarkus-vertx-core:jar:1.11.0.Final:compile
[INFO] | | | +- io.quarkus:quarkus-netty:jar:1.11.0.Final:compile
[INFO] | | | | +- io.netty:netty-codec:jar:4.1.49.Final:compile
[INFO] | | | | \- io.netty:netty-handler:jar:4.1.49.Final:compile
[INFO] | | | \- io.vertx:vertx-core:jar:3.9.5:compile
[INFO] | | | +- io.netty:netty-common:jar:4.1.49.Final:compile
[INFO] | | | +- io.netty:netty-buffer:jar:4.1.49.Final:compile
[INFO] | | | +- io.netty:netty-transport:jar:4.1.49.Final:compile
[INFO] | | | +- io.netty:netty-handler-proxy:jar:4.1.49.Final:compile
[INFO] | | | | \- io.netty:netty-codec-socks:jar:4.1.49.Final:compile
[INFO] | | | +- io.netty:netty-codec-http:jar:4.1.49.Final:compile
[INFO] | | | +- io.netty:netty-codec-http2:jar:4.1.49.Final:compile
[INFO] | | | +- io.netty:netty-resolver:jar:4.1.49.Final:compile
[INFO] | | | +- io.netty:netty-resolver-dns:jar:4.1.49.Final:compile
[INFO] | | | | \- io.netty:netty-codec-dns:jar:4.1.49.Final:compile
[INFO] | | | \- com.fasterxml.jackson.core:jackson-core:jar:2.11.3:compile
[INFO] | | \- io.vertx:vertx-web:jar:3.9.5:compile
[INFO] | | +- io.vertx:vertx-web-common:jar:3.9.5:compile
[INFO] | | +- io.vertx:vertx-auth-common:jar:3.9.5:compile
[INFO] | | \- io.vertx:vertx-bridge-common:jar:3.9.5:compile
[INFO] | \- io.quarkus:quarkus-resteasy-server-common:jar:1.11.0.Final:compile
[INFO] | +- io.quarkus:quarkus-resteasy-common:jar:1.11.0.Final:compile
[INFO] | | +- org.jboss.resteasy:resteasy-core:jar:4.5.8.SP1:compile
[INFO] | | | +- org.jboss.spec.javax.ws.rs:jboss-jaxrs-api_2.1_spec:jar:2.0.1.Final:compile
[INFO] | | | +- org.jboss.spec.javax.xml.bind:jboss-jaxb-api_2.3_spec:jar:2.0.0.Final:compile
[INFO] | | | +- org.jboss.resteasy:resteasy-core-spi:jar:4.5.8.SP1:compile
[INFO] | | | \- com.ibm.async:asyncutil:jar:0.1.0:compile
[INFO] | | \- com.sun.activation:jakarta.activation:jar:1.2.1:compile
[INFO] | \- jakarta.validation:jakarta.validation-api:jar:2.0.2:compile
[INFO] +- io.quarkus:quarkus-junit5:jar:1.11.0.Final:test
[INFO] | +- io.quarkus:quarkus-bootstrap-core:jar:1.11.0.Final:test
[INFO] | | +- io.quarkus:quarkus-bootstrap-app-model:jar:1.11.0.Final:test
[INFO] | | +- io.quarkus:quarkus-bootstrap-maven-resolver:jar:1.11.0.Final:test
[INFO] | | | +- org.apache.maven:maven-embedder:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven:maven-settings:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven:maven-core:jar:3.6.3:test
[INFO] | | | | | +- org.apache.maven:maven-artifact:jar:3.6.3:test
[INFO] | | | | | \- org.codehaus.plexus:plexus-component-annotations:jar:2.1.0:test
[INFO] | | | | +- org.apache.maven:maven-plugin-api:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven:maven-model:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven:maven-model-builder:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven:maven-builder-support:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven.resolver:maven-resolver-api:jar:1.4.1:test
[INFO] | | | | +- org.apache.maven.resolver:maven-resolver-util:jar:1.4.1:test
[INFO] | | | | +- org.apache.maven.shared:maven-shared-utils:jar:3.2.1:test
[INFO] | | | | | \- commons-io:commons-io:jar:2.8.0:test
[INFO] | | | | +- com.google.inject:guice:jar:no_aop:4.2.1:test
[INFO] | | | | | \- com.google.guava:guava:jar:30.1-jre:test
[INFO] | | | | | +- com.google.guava:failureaccess:jar:1.0.1:test
[INFO] | | | | | \- com.google.guava:listenablefuture:jar:9999.0-empty-to-avoid-conflict-with-guava:test
[INFO] | | | | +- org.codehaus.plexus:plexus-utils:jar:3.2.1:test
[INFO] | | | | +- org.codehaus.plexus:plexus-classworlds:jar:2.6.0:test
[INFO] | | | | \- commons-cli:commons-cli:jar:1.4:test
[INFO] | | | +- org.eclipse.sisu:org.eclipse.sisu.plexus:jar:0.3.4:test
[INFO] | | | +- org.apache.maven:maven-settings-builder:jar:3.6.3:test
[INFO] | | | | +- org.codehaus.plexus:plexus-interpolation:jar:1.25:test
[INFO] | | | | \- org.sonatype.plexus:plexus-sec-dispatcher:jar:1.4:test
[INFO] | | | | \- org.sonatype.plexus:plexus-cipher:jar:1.4:test
[INFO] | | | +- org.apache.maven:maven-resolver-provider:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven:maven-repository-metadata:jar:3.6.3:test
[INFO] | | | | +- org.apache.maven.resolver:maven-resolver-spi:jar:1.4.1:test
[INFO] | | | | \- org.apache.maven.resolver:maven-resolver-impl:jar:1.4.1:test
[INFO] | | | +- org.apache.maven.resolver:maven-resolver-connector-basic:jar:1.4.1:test
[INFO] | | | +- org.apache.maven.resolver:maven-resolver-transport-wagon:jar:1.4.1:test
[INFO] | | | +- org.apache.maven.wagon:wagon-http:jar:3.3.4:test
[INFO] | | | | +- org.apache.maven.wagon:wagon-http-shared:jar:3.3.4:test
[INFO] | | | | | \- org.jsoup:jsoup:jar:1.12.1:test
[INFO] | | | | \- org.apache.maven.wagon:wagon-provider-api:jar:3.3.4:test
[INFO] | | | \- org.apache.maven.wagon:wagon-file:jar:3.3.4:test
[INFO] | | +- io.quarkus:quarkus-bootstrap-gradle-resolver:jar:1.11.0.Final:test
[INFO] | | \- io.smallrye.common:smallrye-common-io:jar:1.5.0:compile
[INFO] | +- org.eclipse.sisu:org.eclipse.sisu.inject:jar:0.3.4:test
[INFO] | +- io.quarkus:quarkus-test-common:jar:1.11.0.Final:test
[INFO] | | +- io.quarkus:quarkus-core-deployment:jar:1.11.0.Final:test
[INFO] | | | +- io.quarkus.gizmo:gizmo:jar:1.0.6.Final:test
[INFO] | | | | \- org.ow2.asm:asm-util:jar:9.0:test
[INFO] | | | | +- org.ow2.asm:asm-tree:jar:9.0:test
[INFO] | | | | \- org.ow2.asm:asm-analysis:jar:9.0:test
[INFO] | | | +- org.ow2.asm:asm:jar:9.0:test
[INFO] | | | +- io.quarkus:quarkus-class-change-agent:jar:1.11.0.Final:test
[INFO] | | | +- io.quarkus:quarkus-devtools-utilities:jar:1.11.0.Final:test
[INFO] | | | \- io.quarkus:quarkus-builder:jar:1.11.0.Final:test
[INFO] | | +- io.quarkus:quarkus-jsonp-deployment:jar:1.11.0.Final:test
[INFO] | | | \- io.quarkus:quarkus-jsonp:jar:1.11.0.Final:test
[INFO] | | | \- org.glassfish:jakarta.json:jar:1.1.6:test
[INFO] | | +- org.jboss:jandex:jar:2.2.2.Final:test
[INFO] | | \- org.jboss.logging:commons-logging-jboss-logging:jar:1.0.0.Final:test
[INFO] | +- org.junit.jupiter:junit-jupiter:jar:5.7.0:test
[INFO] | | +- org.junit.jupiter:junit-jupiter-api:jar:5.7.0:test
[INFO] | | | +- org.apiguardian:apiguardian-api:jar:1.1.0:test
[INFO] | | | +- org.opentest4j:opentest4j:jar:1.2.0:test
[INFO] | | | \- org.junit.platform:junit-platform-commons:jar:1.7.0:test
[INFO] | | +- org.junit.jupiter:junit-jupiter-params:jar:5.7.0:test
[INFO] | | \- org.junit.jupiter:junit-jupiter-engine:jar:5.7.0:test
[INFO] | | \- org.junit.platform:junit-platform-engine:jar:1.7.0:test
[INFO] | \- com.thoughtworks.xstream:xstream:jar:1.4.14:test
[INFO] | +- xmlpull:xmlpull:jar:1.1.3.1:test
[INFO] | \- xpp3:xpp3_min:jar:1.1.4c:test
[INFO] \- io.rest-assured:rest-assured:jar:4.3.3:test
[INFO] +- org.codehaus.groovy:groovy:jar:3.0.5:test
[INFO] +- org.codehaus.groovy:groovy-xml:jar:3.0.7:test
[INFO] +- org.apache.httpcomponents:httpclient:jar:4.5.13:test
[INFO] | +- org.apache.httpcomponents:httpcore:jar:4.4.14:test
[INFO] | \- commons-codec:commons-codec:jar:1.14:test
[INFO] +- org.apache.httpcomponents:httpmime:jar:4.5.13:test
[INFO] +- org.hamcrest:hamcrest:jar:2.1:test
[INFO] +- org.ccil.cowan.tagsoup:tagsoup:jar:1.2.1:test
[INFO] +- io.rest-assured:json-path:jar:4.3.3:test
[INFO] | +- org.codehaus.groovy:groovy-json:jar:3.0.5:test
[INFO] | \- io.rest-assured:rest-assured-common:jar:4.3.3:test
[INFO] \- io.rest-assured:xml-path:jar:4.3.3:test
[INFO] +- org.apache.commons:commons-lang3:jar:3.9:test
[INFO] +- jakarta.xml.bind:jakarta.xml.bind-api:jar:2.3.3:test
[INFO] | \- jakarta.activation:jakarta.activation-api:jar:1.2.1:test
[INFO] \- com.sun.xml.bind:jaxb-impl:jar:2.3.3:test
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 6.412 s
[INFO] Finished at: 2021-01-26T15:02:09+08:00
[INFO] ------------------------------------------------------------------------
4.4 The above mvn dependency:tree
display the entire compile, runtime and test dependencies of the application. However, we can use the Maven plugin quarkus-bootstrap-maven-plugin
to display only the runtime dependencies of the Quarkus application.
Put this in pom.xml
<plugin>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-bootstrap-maven-plugin</artifactId>
<version>${quarkus-plugin.version}</version>
</plugin>
In terminal, type mvnw quarkus-bootstrap:build-tree
to display the runtime dependencies.
> mvnw quarkus-bootstrap:build-tree
[INFO] Scanning for projects...
[INFO]
[INFO] ----------------< com.mkyong:quarkus-hello-world-maven >----------------
[INFO] Building quarkus-hello-world-maven 1.0.0-SNAPSHOT
[INFO] --------------------------------[ jar ]---------------------------------
[INFO]
[INFO] --- quarkus-bootstrap-maven-plugin:1.11.0.Final:build-tree (default-cli) @ quarkus-hello-world-maven ---
[INFO] com.mkyong:quarkus-hello-world-maven:pom:1.0.0-SNAPSHOT
[INFO] ├─ io.quarkus:quarkus-arc-deployment:jar:1.11.0.Final (compile)
[INFO] │ ├─ io.quarkus:quarkus-core-deployment:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ org.wildfly.common:wildfly-common:jar:1.5.4.Final-format-001 (compile)
[INFO] │ │ ├─ io.quarkus.gizmo:gizmo:jar:1.0.6.Final (compile)
[INFO] │ │ │ └─ org.ow2.asm:asm-util:jar:9.0 (compile)
[INFO] │ │ │ ├─ org.ow2.asm:asm-tree:jar:9.0 (compile)
[INFO] │ │ │ └─ org.ow2.asm:asm-analysis:jar:9.0 (compile)
[INFO] │ │ ├─ org.jboss:jandex:jar:2.2.2.Final (compile)
[INFO] │ │ ├─ org.ow2.asm:asm:jar:9.0 (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-development-mode-spi:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-class-change-agent:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-bootstrap-core:jar:1.11.0.Final (compile)
[INFO] │ │ │ ├─ io.quarkus:quarkus-bootstrap-app-model:jar:1.11.0.Final (compile)
[INFO] │ │ │ │ └─ org.jboss.logging:commons-logging-jboss-logging:jar:1.0.0.Final (runtime)
[INFO] │ │ │ ├─ io.quarkus:quarkus-bootstrap-maven-resolver:jar:1.11.0.Final (compile)
[INFO] │ │ │ │ ├─ org.apache.maven:maven-embedder:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven:maven-settings:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven:maven-core:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ │ ├─ org.apache.maven:maven-artifact:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ │ └─ org.codehaus.plexus:plexus-component-annotations:jar:2.1.0 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven:maven-plugin-api:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven:maven-model:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven:maven-model-builder:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven:maven-builder-support:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven.resolver:maven-resolver-api:jar:1.4.1 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven.resolver:maven-resolver-util:jar:1.4.1 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven.shared:maven-shared-utils:jar:3.2.1 (compile)
[INFO] │ │ │ │ │ │ └─ commons-io:commons-io:jar:2.8.0 (compile)
[INFO] │ │ │ │ │ ├─ com.google.inject:guice:jar:no_aop:4.2.1 (compile)
[INFO] │ │ │ │ │ │ └─ com.google.guava:guava:jar:30.1-jre (compile)
[INFO] │ │ │ │ │ │ ├─ com.google.guava:failureaccess:jar:1.0.1 (compile)
[INFO] │ │ │ │ │ │ └─ com.google.guava:listenablefuture:jar:9999.0-empty-to-avoid-conflict-with-guava (compile)
[INFO] │ │ │ │ │ ├─ org.codehaus.plexus:plexus-utils:jar:3.2.1 (compile)
[INFO] │ │ │ │ │ ├─ org.codehaus.plexus:plexus-classworlds:jar:2.6.0 (compile)
[INFO] │ │ │ │ │ ├─ commons-cli:commons-cli:jar:1.4 (compile)
[INFO] │ │ │ │ │ └─ org.apache.commons:commons-lang3:jar:3.9 (compile)
[INFO] │ │ │ │ ├─ org.eclipse.sisu:org.eclipse.sisu.plexus:jar:0.3.4 (compile)
[INFO] │ │ │ │ ├─ org.apache.maven:maven-settings-builder:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.codehaus.plexus:plexus-interpolation:jar:1.25 (compile)
[INFO] │ │ │ │ │ └─ org.sonatype.plexus:plexus-sec-dispatcher:jar:1.4 (compile)
[INFO] │ │ │ │ │ └─ org.sonatype.plexus:plexus-cipher:jar:1.4 (compile)
[INFO] │ │ │ │ ├─ org.apache.maven:maven-resolver-provider:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven:maven-repository-metadata:jar:3.6.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven.resolver:maven-resolver-spi:jar:1.4.1 (compile)
[INFO] │ │ │ │ │ └─ org.apache.maven.resolver:maven-resolver-impl:jar:1.4.1 (compile)
[INFO] │ │ │ │ ├─ org.apache.maven.resolver:maven-resolver-connector-basic:jar:1.4.1 (compile)
[INFO] │ │ │ │ ├─ org.apache.maven.resolver:maven-resolver-transport-wagon:jar:1.4.1 (compile)
[INFO] │ │ │ │ ├─ org.apache.maven.wagon:wagon-http:jar:3.3.4 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.maven.wagon:wagon-http-shared:jar:3.3.4 (compile)
[INFO] │ │ │ │ │ │ └─ org.jsoup:jsoup:jar:1.11.3 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.httpcomponents:httpclient:jar:4.5.13 (compile)
[INFO] │ │ │ │ │ │ └─ commons-codec:commons-codec:jar:1.14 (compile)
[INFO] │ │ │ │ │ ├─ org.apache.httpcomponents:httpcore:jar:4.4.14 (compile)
[INFO] │ │ │ │ │ └─ org.apache.maven.wagon:wagon-provider-api:jar:3.3.3 (compile)
[INFO] │ │ │ │ └─ org.apache.maven.wagon:wagon-file:jar:3.3.4 (compile)
[INFO] │ │ │ ├─ io.quarkus:quarkus-bootstrap-gradle-resolver:jar:1.11.0.Final (compile)
[INFO] │ │ │ └─ io.smallrye.common:smallrye-common-io:jar:1.5.0 (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-devtools-utilities:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ org.eclipse.sisu:org.eclipse.sisu.inject:jar:0.3.4 (runtime)
[INFO] │ │ ├─ io.quarkus:quarkus-core:jar:1.11.0.Final (compile)
[INFO] │ │ │ ├─ jakarta.inject:jakarta.inject-api:jar:1.0 (compile)
[INFO] │ │ │ ├─ io.quarkus:quarkus-ide-launcher:jar:1.11.0.Final (compile)
[INFO] │ │ │ ├─ io.smallrye.config:smallrye-config:jar:1.10.0 (compile)
[INFO] │ │ │ │ ├─ io.smallrye.common:smallrye-common-annotation:jar:1.5.0 (compile)
[INFO] │ │ │ │ ├─ io.smallrye.config:smallrye-config-common:jar:1.10.0 (compile)
[INFO] │ │ │ │ │ └─ org.eclipse.microprofile.config:microprofile-config-api:jar:1.4 (compile)
[INFO] │ │ │ │ ├─ io.smallrye.common:smallrye-common-expression:jar:1.5.0 (compile)
[INFO] │ │ │ │ │ └─ io.smallrye.common:smallrye-common-function:jar:1.5.0 (compile)
[INFO] │ │ │ │ ├─ io.smallrye.common:smallrye-common-constraint:jar:1.5.0 (compile)
[INFO] │ │ │ │ └─ io.smallrye.common:smallrye-common-classloader:jar:1.5.0 (compile)
[INFO] │ │ │ ├─ org.jboss.logmanager:jboss-logmanager-embedded:jar:1.0.6 (compile)
[INFO] │ │ │ ├─ org.jboss.logging:jboss-logging-annotations:jar:2.2.0.Final (compile)
[INFO] │ │ │ ├─ org.jboss.threads:jboss-threads:jar:3.2.0.Final (compile)
[INFO] │ │ │ ├─ org.slf4j:slf4j-api:jar:1.7.30 (compile)
[INFO] │ │ │ ├─ org.jboss.slf4j:slf4j-jboss-logmanager:jar:1.1.0.Final (compile)
[INFO] │ │ │ └─ io.quarkus:quarkus-bootstrap-runner:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-builder:jar:1.11.0.Final (compile)
[INFO] │ │ └─ org.graalvm.sdk:graal-sdk:jar:20.3.0 (compile)
[INFO] │ ├─ io.quarkus:quarkus-vertx-http-dev-console-spi:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-vertx-http-dev-console-runtime-spi:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus.arc:arc:jar:1.11.0.Final (compile)
[INFO] │ │ │ └─ jakarta.transaction:jakarta.transaction-api:jar:1.3.3 (compile)
[INFO] │ │ └─ io.vertx:vertx-web:jar:3.9.5 (compile)
[INFO] │ │ ├─ io.vertx:vertx-web-common:jar:3.9.5 (compile)
[INFO] │ │ ├─ io.vertx:vertx-auth-common:jar:3.9.5 (compile)
[INFO] │ │ ├─ io.vertx:vertx-bridge-common:jar:3.9.5 (compile)
[INFO] │ │ └─ io.vertx:vertx-core:jar:3.9.5 (compile)
[INFO] │ │ ├─ io.netty:netty-common:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-buffer:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-transport:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-handler:jar:4.1.49.Final (compile)
[INFO] │ │ │ └─ io.netty:netty-codec:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-handler-proxy:jar:4.1.49.Final (compile)
[INFO] │ │ │ └─ io.netty:netty-codec-socks:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-codec-http:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-codec-http2:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-resolver:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ io.netty:netty-resolver-dns:jar:4.1.49.Final (compile)
[INFO] │ │ │ └─ io.netty:netty-codec-dns:jar:4.1.49.Final (compile)
[INFO] │ │ ├─ com.fasterxml.jackson.core:jackson-core:jar:2.11.3 (compile)
[INFO] │ │ └─ com.fasterxml.jackson.core:jackson-databind:jar:2.11.3 (compile)
[INFO] │ │ └─ com.fasterxml.jackson.core:jackson-annotations:jar:2.11.3 (compile)
[INFO] │ ├─ io.quarkus:quarkus-arc:jar:1.11.0.Final (compile)
[INFO] │ │ └─ org.eclipse.microprofile.context-propagation:microprofile-context-propagation-api:jar:1.0.1 (compile)
[INFO] │ └─ io.quarkus.arc:arc-processor:jar:1.11.0.Final (compile)
[INFO] │ ├─ jakarta.enterprise:jakarta.enterprise.cdi-api:jar:2.0.2 (compile)
[INFO] │ │ ├─ jakarta.el:jakarta.el-api:jar:3.0.3 (compile)
[INFO] │ │ └─ jakarta.interceptor:jakarta.interceptor-api:jar:1.2.5 (compile)
[INFO] │ ├─ org.jboss.logging:jboss-logging:jar:3.4.1.Final (compile)
[INFO] │ └─ jakarta.annotation:jakarta.annotation-api:jar:1.3.5 (compile)
[INFO] └─ io.quarkus:quarkus-resteasy-deployment:jar:1.11.0.Final (compile)
[INFO] ├─ io.quarkus:quarkus-resteasy-server-common-deployment:jar:1.11.0.Final (compile)
[INFO] │ ├─ io.quarkus:quarkus-resteasy-common-deployment:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-resteasy-common:jar:1.11.0.Final (compile)
[INFO] │ │ │ ├─ org.jboss.resteasy:resteasy-core:jar:4.5.8.SP1 (compile)
[INFO] │ │ │ │ ├─ org.jboss.spec.javax.ws.rs:jboss-jaxrs-api_2.1_spec:jar:2.0.1.Final (compile)
[INFO] │ │ │ │ ├─ org.jboss.spec.javax.xml.bind:jboss-jaxb-api_2.3_spec:jar:2.0.0.Final (compile)
[INFO] │ │ │ │ ├─ org.jboss.resteasy:resteasy-core-spi:jar:4.5.8.SP1 (compile)
[INFO] │ │ │ │ └─ com.ibm.async:asyncutil:jar:0.1.0 (compile)
[INFO] │ │ │ └─ com.sun.activation:jakarta.activation:jar:1.2.1 (compile)
[INFO] │ │ └─ io.quarkus:quarkus-resteasy-common-spi:jar:1.11.0.Final (compile)
[INFO] │ ├─ io.quarkus:quarkus-resteasy-server-common-spi:jar:1.11.0.Final (compile)
[INFO] │ └─ io.quarkus:quarkus-resteasy-server-common:jar:1.11.0.Final (compile)
[INFO] │ └─ jakarta.validation:jakarta.validation-api:jar:2.0.2 (compile)
[INFO] ├─ io.quarkus:quarkus-undertow-spi:jar:1.11.0.Final (compile)
[INFO] │ ├─ io.quarkus.http:quarkus-http-servlet:jar:3.0.18.Final (compile)
[INFO] │ │ └─ io.quarkus.http:quarkus-http-core:jar:3.0.18.Final (compile)
[INFO] │ │ └─ io.quarkus.http:quarkus-http-http-core:jar:3.0.18.Final (compile)
[INFO] │ ├─ jakarta.servlet:jakarta.servlet-api:jar:4.0.3 (compile)
[INFO] │ └─ org.jboss.metadata:jboss-metadata-web:jar:11.0.0.Final (compile)
[INFO] │ └─ org.jboss.metadata:jboss-metadata-common:jar:11.0.0.Final (compile)
[INFO] ├─ io.quarkus:quarkus-vertx-http-deployment:jar:1.11.0.Final (compile)
[INFO] │ ├─ io.quarkus:quarkus-vertx-core-deployment:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-netty-deployment:jar:1.11.0.Final (compile)
[INFO] │ │ │ └─ io.quarkus:quarkus-netty:jar:1.11.0.Final (compile)
[INFO] │ │ └─ io.quarkus:quarkus-vertx-core:jar:1.11.0.Final (compile)
[INFO] │ ├─ io.quarkus:quarkus-vertx-http:jar:1.11.0.Final (compile)
[INFO] │ │ ├─ io.quarkus:quarkus-security-runtime-spi:jar:1.11.0.Final (compile)
[INFO] │ │ └─ io.quarkus.security:quarkus-security:jar:1.1.3.Final (compile)
[INFO] │ ├─ io.quarkus:quarkus-kubernetes-spi:jar:1.11.0.Final (compile)
[INFO] │ ├─ io.quarkus.qute:qute-core:jar:1.11.0.Final (compile)
[INFO] │ │ └─ io.smallrye.reactive:mutiny:jar:0.12.5 (compile)
[INFO] │ │ └─ org.reactivestreams:reactive-streams:jar:1.0.3 (compile)
[INFO] │ └─ org.yaml:snakeyaml:jar:1.27 (compile)
[INFO] ├─ io.quarkus:quarkus-resteasy:jar:1.11.0.Final (compile)
[INFO] └─ io.quarkus:quarkus-security-spi:jar:1.11.0.Final (compile)
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 3.256 s
[INFO] Finished at: 2021-01-27T21:27:24+08:00
[INFO] ------------------------------------------------------------------------
P.S Types mvnw quarkus-bootstrap:help
to list out all the available goals.
5. Quarkus JAX-RX endpoint
The quarkus-maven-plugin
generates a simple HelloResource.java
for the /hello
endpoint and also associate unit test.
5.1 A simple JAX-RX endpoint /hello
, and return a plain text output "Hello RESTEasy".
package com.mkyong.quarkus;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class HelloResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello RESTEasy";
}
}
5.2 A unit test to test the above endpoint.
package com.mkyong.quarkus;
import io.quarkus.test.junit.QuarkusTest;
import org.junit.jupiter.api.Test;
import static io.restassured.RestAssured.given;
import static org.hamcrest.CoreMatchers.is;
@QuarkusTest
public class HelloResourceTest {
@Test
public void testHelloEndpoint() {
given()
.when().get("/hello")
.then()
.statusCode(200)
.body(is("Hello RESTEasy"));
}
}
6. Quarkus and Dockerfile
The quarkus-maven-plugin
also generates three Dockerfile
files to build a Docker container for the JAR file or the native executable.
Dockerfile.fast-jar
– Build a docker container for the JAR file, this is a new packaging format (Fast-jar) since Quarkus 1.5 released, for faster startup times (likely default format in the future release).Dockerfile.jvm
– Build a docker container for the JAR file.Dockerfile.native
– Build a docker container for native executable.
7. Run Quarkus in Dev Mode
7.1 In a separated terminal, type command mvnw compile quarkus:dev
to run Quarkus application in development mode, and by default, enabling hot deployment with background compilation, which means if we modify some code, the changes will automatically take effect.
By default, the Quarkus application starts at port 8080
.
> mvnw compile quarkus:dev
OpenJDK 64-Bit Server VM warning: forcing TieredStopAtLevel to full optimization because JVMCI is enabled
Listening for transport dt_socket at address: 5005
__ ____ __ _____ ___ __ ____ ______
--/ __ \/ / / / _ | / _ \/ //_/ / / / __/
-/ /_/ / /_/ / __ |/ , _/ ,< / /_/ /\ \
--\___\_\____/_/ |_/_/|_/_/|_|\____/___/
2021-01-26 16:19:28,903 INFO [io.quarkus] (Quarkus Main Thread) quarkus-hello-world-maven 1.0.0-SNAPSHOT
on JVM (powered by Quarkus 1.11.0.Final) started in 1.677s. Listening on: http://localhost:8080
2021-01-26 16:19:28,905 INFO [io.quarkus] (Quarkus Main Thread) Profile dev activated. Live Coding activated.
2021-01-26 16:19:28,912 INFO [io.quarkus] (Quarkus Main Thread) Installed features: [cdi, resteasy]
7.2 Access the /hello
endpoint.
> curl http://localhost:8080/hello
Hello RESTEasy
7.3 We modify the /hello
endpoint to return a new string "Hello World Quarkus", and save it.
package com.mkyong.quarkus;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class HelloResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
//return "Hello RESTEasy";
return "Hello World Quarkus";
}
}
7.4 Back to the terminal that starts the Quarkus application, the vert.x-worker-thread
will detect the changed file and do hot replace automatically.
OpenJDK 64-Bit Server VM warning: forcing TieredStopAtLevel to full optimization because JVMCI is enabled
Listening for transport dt_socket at address: 5005
__ ____ __ _____ ___ __ ____ ______
--/ __ \/ / / / _ | / _ \/ //_/ / / / __/
-/ /_/ / /_/ / __ |/ , _/ ,< / /_/ /\ \
--\___\_\____/_/ |_/_/|_/_/|_|\____/___/
2021-01-26 16:19:28,903 INFO [io.quarkus] (Quarkus Main Thread) quarkus-hello-world-maven 1.0.0-SNAPSHOT
on JVM (powered by Quarkus 1.11.0.Final) started in 1.677s. Listening on: http://localhost:8080
2021-01-26 16:19:28,905 INFO [io.quarkus] (Quarkus Main Thread) Profile dev activated. Live Coding activated.
2021-01-26 16:19:28,912 INFO [io.quarkus] (Quarkus Main Thread) Installed features: [cdi, resteasy]
2021-01-26 21:52:36,449 INFO [io.qua.dep.dev.RuntimeUpdatesProcessor] (vert.x-worker-thread-1) Changed source files detected,
recompiling [C:\Users\mkyong\projects\quarkus\quarkus-hello-world-maven\src\main\java\com\mkyong\quarkus\HelloResource.java]
2021-01-26 21:52:36,940 INFO [io.qua.dep.dev.RuntimeUpdatesProcessor] (vert.x-worker-thread-1) Application restart not required, replacing classes via instrumentation
2021-01-26 21:52:36,972 INFO [io.qua.dep.dev.RuntimeUpdatesProcessor] (vert.x-worker-thread-1) Hot replace performed via instrumentation, no restart needed, total time: 0.525s
7.4 Access the /hello
endpoint again to see the new changes.
> curl http://localhost:8080/hello
Hello World Quarkus
7.5 CTRL + C to stop the running Quarkus application.
Further Reading
Download Source Code
$ git clone https://github.com/mkyong/quarkus
$ cd quarkus-hello-world-maven
$ mvnw compile quarkus:dev
$ curl http://localhost:8080/hello
There is a typo in the first title, it should read
quarkus
notquartus
please fix this typo.Thank you for the tutorial!