Check if element exists in JavaScript
In JavaScript, we can use document.querySelector()
to check if an element exists. The document.querySelector()
searches for and returns the first element matching a selector or set of selectors. If it finds no matching elements, it returns null.
For example, to check if an element with the id elementId
exists:
if (document.querySelector("#elementId")) {
// The element exists
} else {
// The element does not exist
}
For example, to check if an element with the class .elementClass
exists:
if (document.querySelector(".elementClass")) {
// The element exists
} else {
// The element does not exist
}
Check if an element exists in JavaScript
Below is a JavaScript example to show the use of document.querySelector()
to check if a specific element exists.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript check elements exists</title>
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', function() {
if (document.querySelector("#title")) {
console.log("The element exists");
} else {
console.log("The element does not exist");
}
if (document.querySelector(".error")) {
console.log("The element exists");
} else {
console.log("The element does not exist");
}
if (document.querySelector("#abc")) {
console.log("The element exists");
} else {
console.log("The element does not exist");
}
});
</script>
</head>
<body>
<div id="title">This is title</div>
<div class="error">This is error</div>
</body>
</html>
Output
The element exists
The element exists
The element does not exist
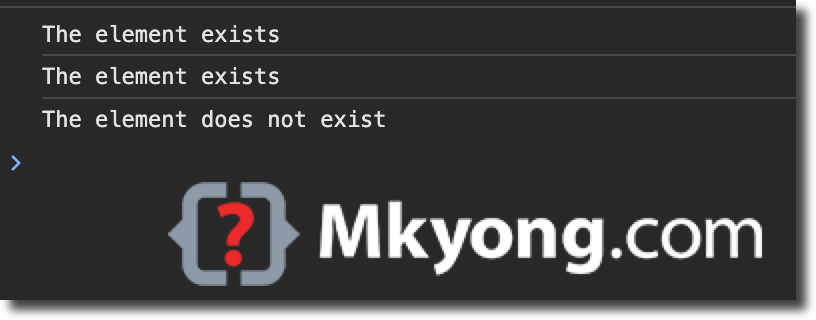
References
About Author
Comments
Subscribe
0 Comments