JavaFX Transformations Examples
This article will show you different transformations in JavaFX and how to use them. In JavaFX, we use transformations to translate (moves), scale, rotate and shear the nodes.
Tested with
- Java 17
- JavaFX 17.0.6
- Maven 3
Table of Contents
- 1. JavaFX Transformations example
- 2. Translation Transformation
- 3. Rotation Transformation
- 4. Scale Transformation
- 5. Shear Transformation
- 6. Combining Transformations
- 7. Download Source Code
- 8. References
1. JavaFX Transformations example
Below is the code to show the common JavaFX transformations.
TransformationApp.java
package com.mkyong.javafx;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.transform.Rotate;
import javafx.scene.transform.Scale;
import javafx.scene.transform.Shear;
import javafx.stage.Stage;
public class TransformationApp extends Application {
public static void main(String[] args) {
launch();
}
private void transform(Rectangle box) {
// playground here.
}
@Override
public void start(Stage primaryStage) throws Exception {
// box with 200 width and 100 height, in color red
Rectangle box = new Rectangle(200, 100, Color.RED);
transform(box);
Scene scene = new Scene(new Pane(box), 640, 480, Color.WHITE);
primaryStage.setScene(scene);
primaryStage.setTitle("JavaFX Transformations examples");
primaryStage.show();
}
}
Output
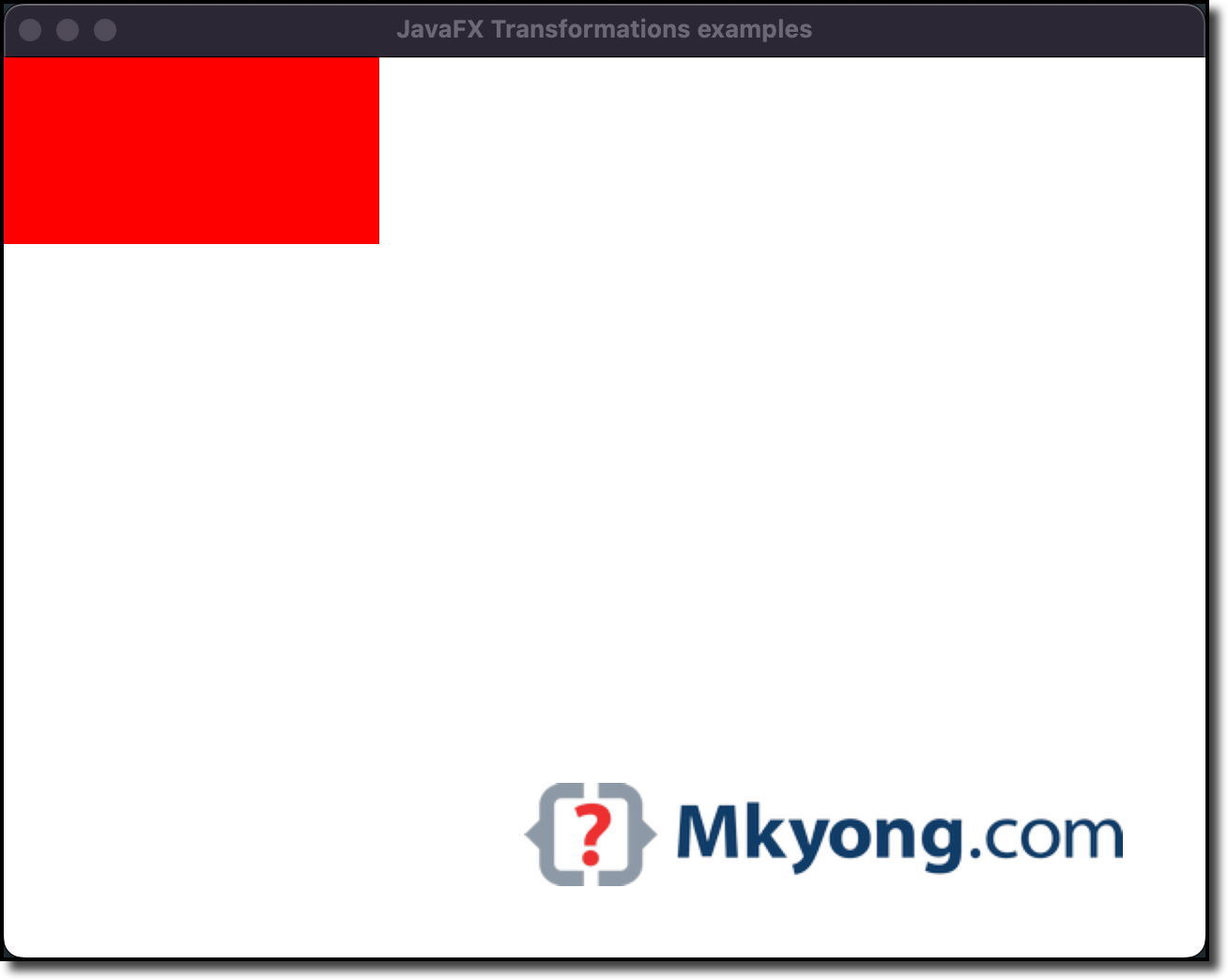
2. Translation Transformation
In JavaFX, the translate
means moving a node from its original position to a new position. For example, the below code moves a node 100 pixels to the right and 150 pixels down.
TransformationApp.java
private void transform(Rectangle box) {
// Translate
// translate means moves,
// below moves the box 100 pixels to the right and 150 pixels down.
box.setTranslateX(100);
box.setTranslateY(150);
}
Output
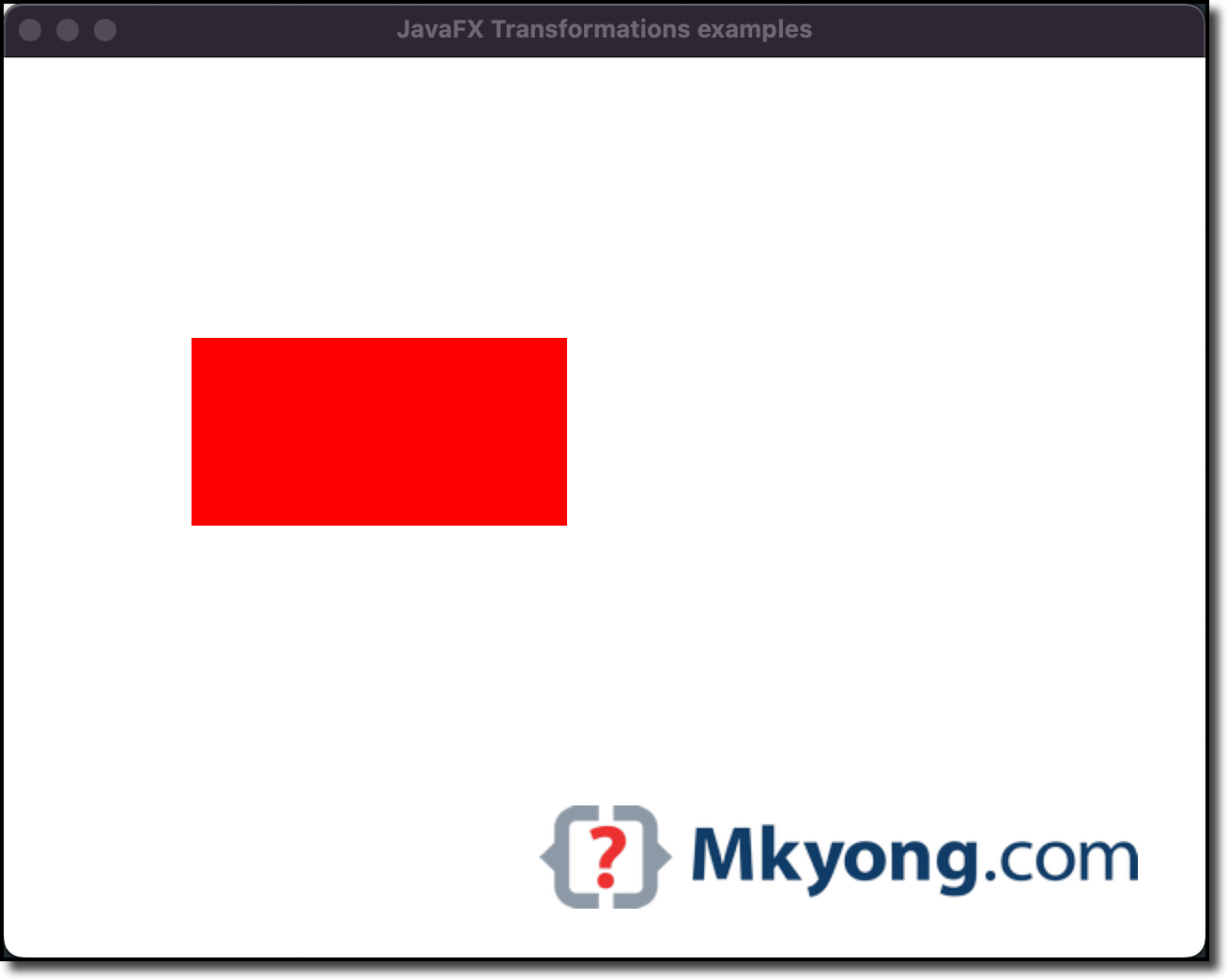
3. Rotation Transformation
The below code rotates a node 45 degrees around its center.
TransformationApp.java
private void transform(Rectangle box) {
box.setTranslateX(100);
box.setTranslateY(150);
// Alternatively, can rotate like this
// new Rotate(angle, pivotX, pivotY)
// Rotate rotate = new Rotate(45, box.getWidth() / 2, box.getHeight() / 2);
// box.getTransforms().add(rotate);
// Let’s rotate the box by 45 degrees.
box.setRotate(45);
}
Output
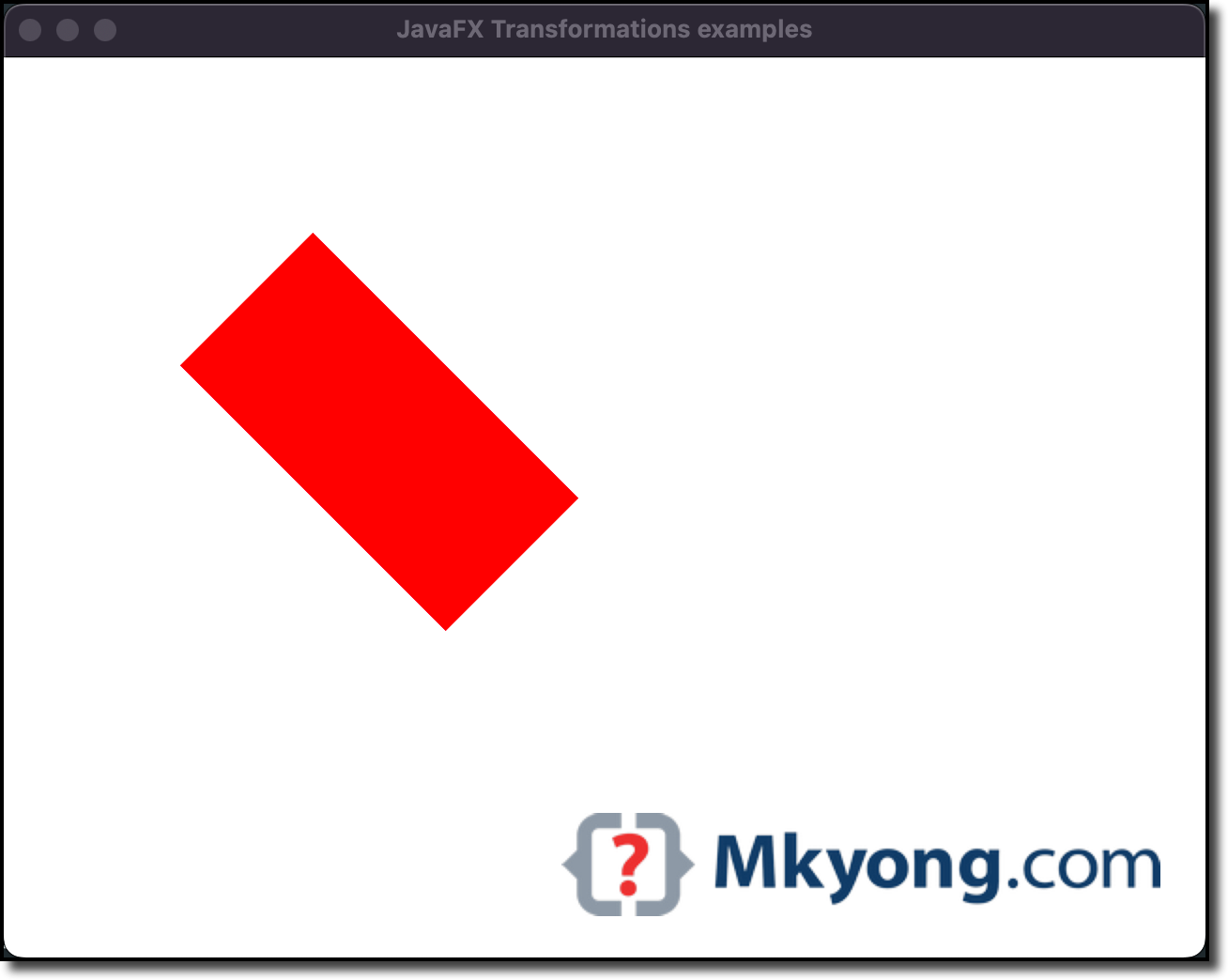
4. Scale Transformation
The below code resizes a node by scaling factors in the x and y directions.
TransformationApp.java
private void transform(Rectangle box) {
box.setTranslateX(100);
box.setTranslateY(150);
// Scale
box.setScaleX(1.5);
box.setScaleY(1.5);
}
Output
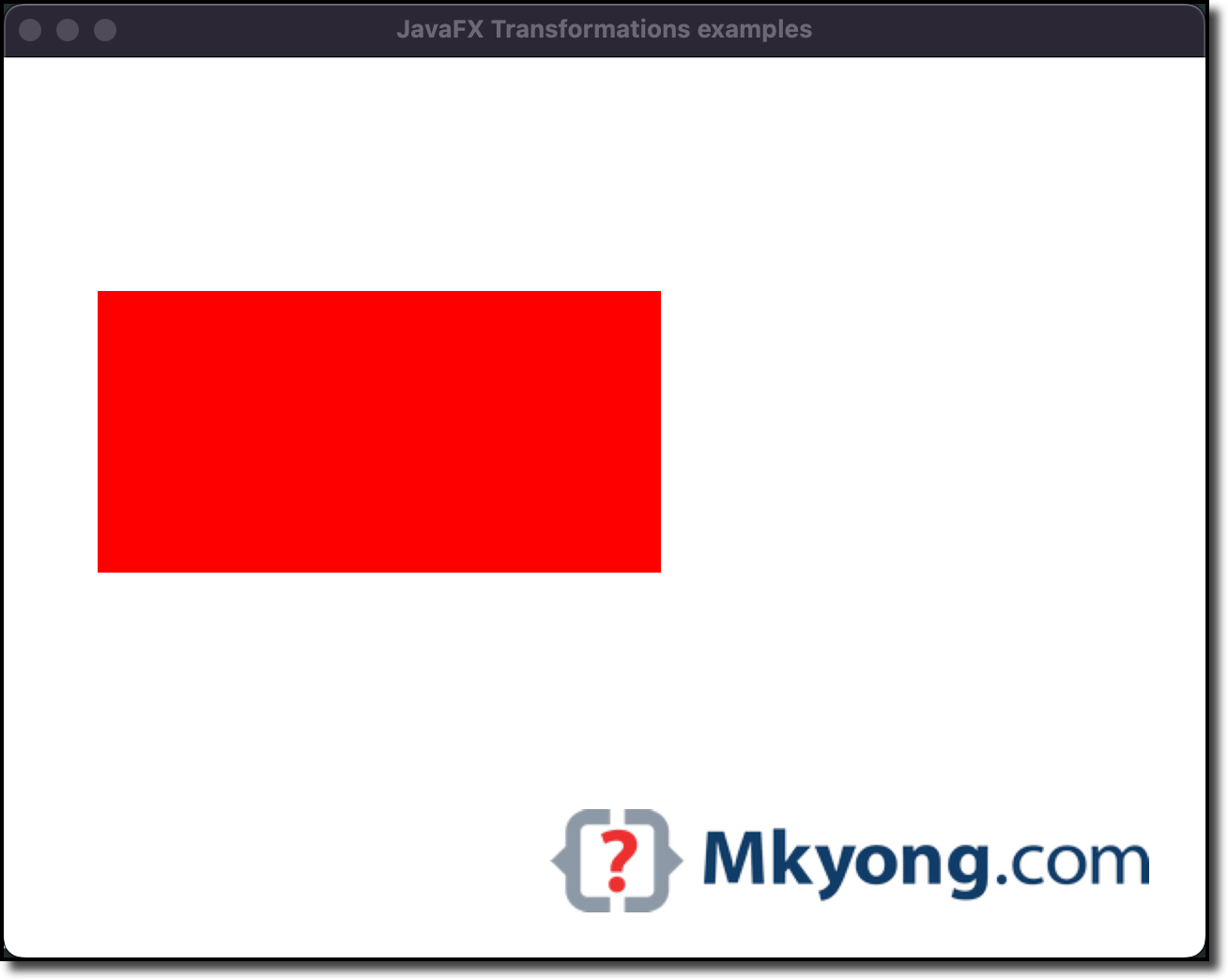
5. Shear Transformation
The below code slant a node along the x-axis.
TransformationApp.java
private void transform(Rectangle box) {
box.setTranslateX(100);
box.setTranslateY(150);
// Shear
// slants a box
box.getTransforms().add(new Shear(0,0.5));
}
Output
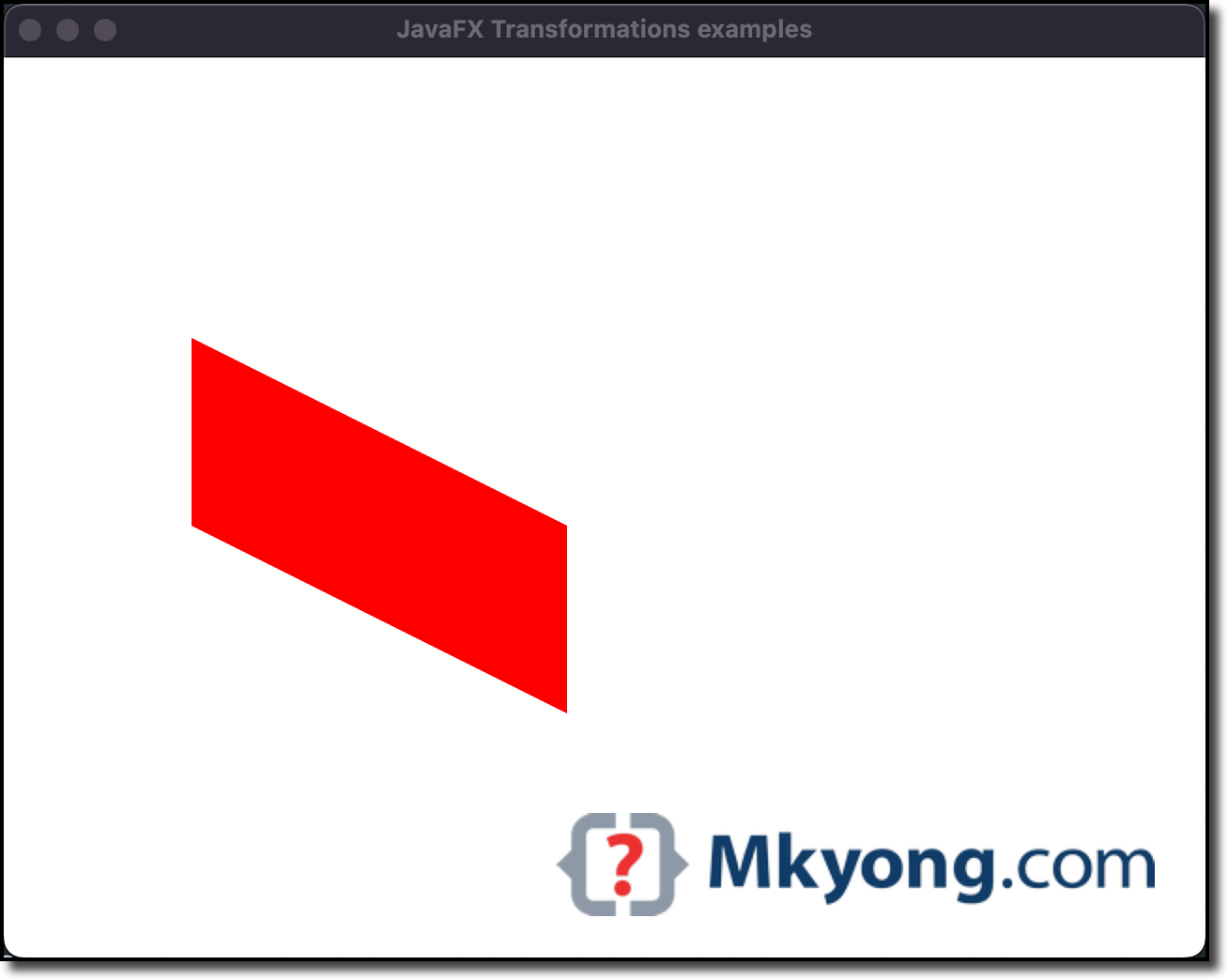
6. Combining Transformations
The below code applies multiple transformations (rotate, scale, and shear) to a node.
TransformationApp.java
private void transform(Rectangle box) {
box.setTranslateX(100);
box.setTranslateY(150);
// combining transformations
box.getTransforms().addAll(
new Rotate(90, box.getWidth() / 2, box.getHeight() / 2),
new Scale(1.2, 1.2),
new Shear(0.5, 0));
}
Output
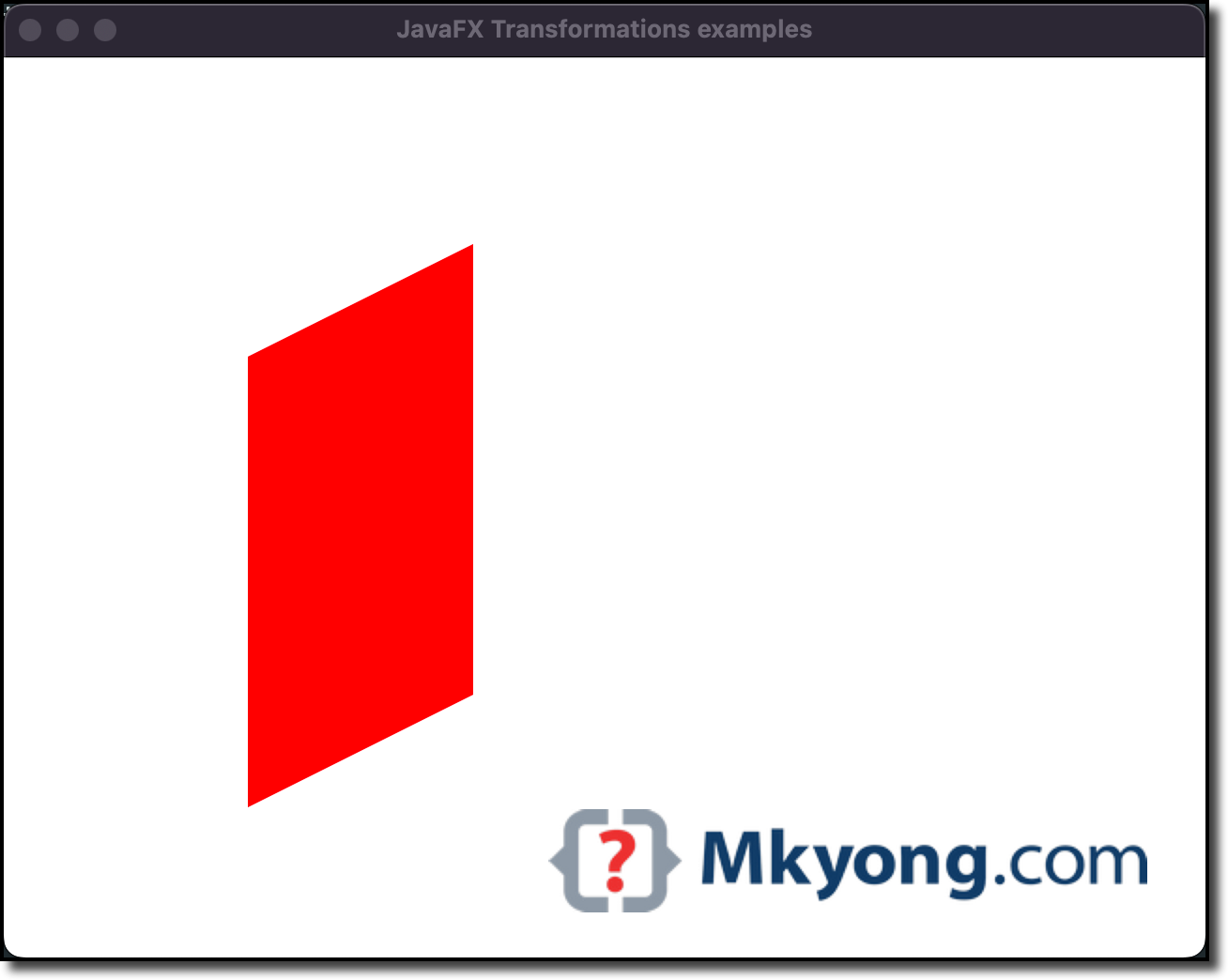
7. Download Source Code
8. References
About Author
Comments
Subscribe
4 Comments
Most Voted