What is new in Java 12
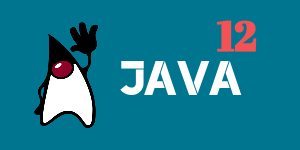
Java 12 reached General Availability on 19 March 2019, download Java 12 here or this openJDK archived.
Java 12 features.
- 1. JEP 189: Shenandoah: A Low-Pause-Time Garbage Collector (Experimental)
- 2. JEP 230: Microbenchmark Suite
- 3. JEP 325: Switch Expressions (Preview)
- 4. JEP 334: JVM Constants API
- 5. JEP 340: One AArch64 Port, Not Two
- 6. JEP 341: Default CDS Archives
- 7. JEP 344: Abortable Mixed Collections for G1
- 8. JEP 346: Promptly Return Unused Committed Memory from G1
Java 12 developer features.
Switch expression (preview)
1. JEP 189: Shenandoah: A Low-Pause-Time Garbage Collector (Experimental)
Shenandoah is a new low-pause and concurrent garbage collector, read this research paper, it reduces GC pause time and independent of the Java heap size (5M or 5G of heap size have the same pause time, useful for large heap applications.)
This GC is an experimental feature, and we need to use the following options to enable the new Shenandoah GC.
-XX:+UnlockExperimentalVMOptions -XX:+UseShenandoahGC
However, both Oracle JDK and OpenJDK don’t contain this new Shenandoah GC, read this also Not all OpenJDK 12 builds include Shenandoah: Here’s why.
C:\Users\mkyong> java -version
java version "12" 2019-03-19
Java(TM) SE Runtime Environment (build 12+33)
Java HotSpot(TM) 64-Bit Server VM (build 12+33, mixed mode, sharing)
C:\Users\mkyong> java -XX:+UnlockExperimentalVMOptions -XX:+UseShenandoahGC
Error occurred during initialization of VM
Option -XX:+UseShenandoahGC not supported
To try Shenandoah GC, we need other JDK build like AdoptOpenJDK.
P.S This Shenandoah GC became a product feature in Java 15 JEP 379.
Further Reading
2. JEP 230: Microbenchmark Suite
Added a range of Java Microbenchmark Harness (JMH) benchmarks to the JDK source code, for those interested to add or modify the JDK source code itself, now they have a way to compare the performance.
Note
Sorry, not sure how it works, please comment below if you know how to run the JDK’s benchmark tests.
Further Reading
3. JEP 325: Switch Expressions (Preview)
This JEP enhanced the existing switch statements (returns nothing) to support switch expressions (returns something).
Traditional switch statements, we can return a value by assigning the value to a variable:
private static String getText(int number) {
String result = "";
switch (number) {
case 1, 2:
result = "one or two";
break;
case 3:
result = "three";
break;
case 4, 5, 6:
result = "four or five or six";
break;
default:
result = "unknown";
break;
};
return result;
}
In Java 12, we can use break
or case L ->
to return a value from a switch.
private static String getText(int number) {
String result = switch (number) {
case 1, 2:
break "one or two";
case 3:
break "three";
case 4, 5, 6:
break "four or five or six";
default:
break "unknown";
};
return result;
}
case L ->
syntax.
private static String getText(int number) {
return switch (number) {
case 1, 2 -> "one or two";
case 3 -> "three";
case 4, 5, 6 -> "four or five or six";
default -> "unknown";
};
}
Note
This switch expressions have a second preview in Java 13 (dropped the break
in favor of yield
), and this switch expressions became a standard feature in Java 14.
Futher Reading
To enable the Java 12 preview features:
javac --enable-preview --release 12 Example.java
java --enable-preview Example
4. JEP 334: JVM Constants API
A new package java.lang.constant
, a list of new classes and interfaces to model the key class-file and run-time artifacts, for example, the constant pool.
Further Reading
5. JEP 340: One AArch64 Port, Not Two
Before Java 12, there are two different source code or ports for the 64-bit ARM architecture.
- Oracle –
src/hotspot/cpu/arm
- Red Hat? –
src/hotspot/cpu/aarch64
Java 12 removed the Oracle src/hotspot/cpu/arm
port, and maintain only one port src/hotspot/cpu/aarch64
, and make this aarch64
the default build for the 64-bit ARM architecture.
Further Reading
6. JEP 341: Default CDS Archives
The Class Data Sharing (CDS) improved the start-up time by reuse an existing archive file.
Before Java 12, we need to use -Xshare: dump
to generate the CDS archive file for the JDK classes. In Java 12, there is a new classes.jsa
file in the /bin/server/
directory, a default CDS archive file for the JDK classes.
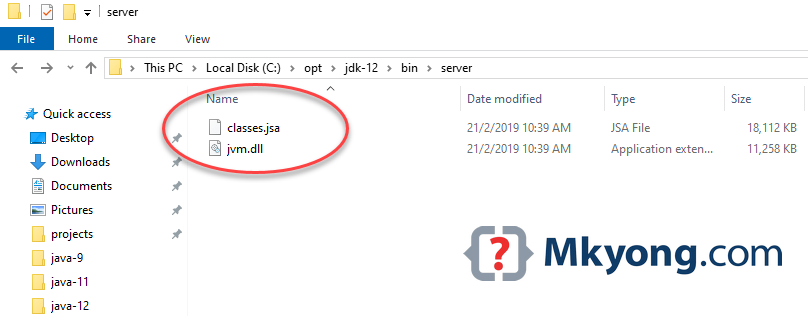
Further Reading
7. JEP 344: Abortable Mixed Collections for G1
This JEP improves the performance of the Garbage-first (G1) collector, by splits the problematic collection set into two parts – mandatory and optional. The G1 will abort the optional part if lack of time to handle it.
Further Reading
8. JEP 346: Promptly Return Unused Committed Memory from G1
This JEP improves the performance of the Garbage-first (G1) collector. If the application is low of the activity or idle, G1 periodically trigger a concurrent cycle to determine overall Java heap usage and return unused Java heap memory to the operating system.
Further Reading
Download Source Code
$ git clone https://github.com/mkyong/core-java
$ cd java-12
References
- OpenJDK 12 Project
- Java version history
- Shenandoah OpenJDK Home
- Shenandoah research paper
- Java 12 Switch expressions
- Java 13 Switch Expressions
- Java Microbenchmark Harness (JMH)
- java.lang.constant
- ARM architecture
- Class Data Sharing
- 39 New Features (and APIs) in JDK 12
- Wikipedia – Garbage-first collector
- Getting Started with the G1 Garbage Collector
- What’s New in Java Security?
Good and crisp presentation of java 12 features, on a high level – Simple!